OEColor¶
class OEColor
The OEColor class represents colors in terms of
RGBA (red, green, blue, alpha) components, where alpha is used as an
opacity channel. Each color component is represented by one byte in the
range of 0
to 255
. Meaning that
\(2^8*2^8*2^8 = 16,777,216\) different colors can be
represented.
The value of alpha is also in the range of 0
to 255
. If a
color has an alpha value of 0
, then the color is fully
transparent, i.e., invisible. If a color has an alpha value
255
, then the color is fully opaque.
OESystem provides many predefined colors. For example, the color
black is represented by
OEBlack
(R=0, G=0, B=0),
and the color white is represented by
OEWhite
(R=255, G=255,
B=255). The full list of pre-defined colors is shown in
Figure: List of pre-defined colors
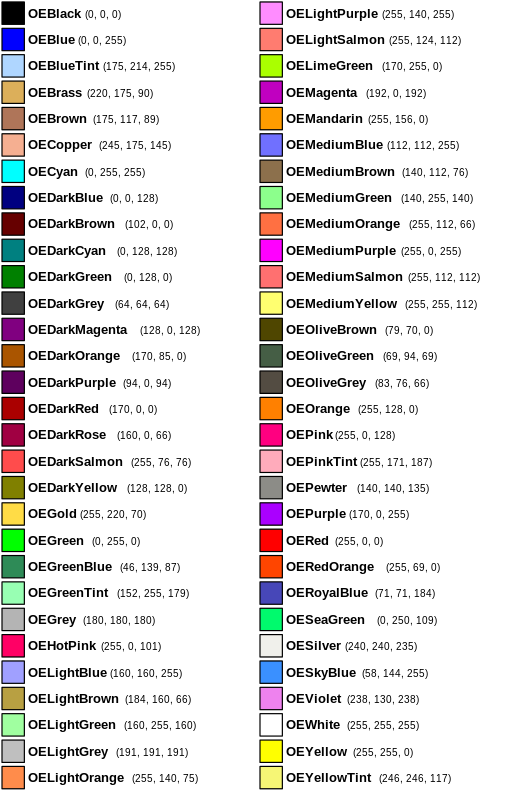
List of pre-defined colors¶
Note
The alpha for all pre-defined colors is 255
, entirely
opaque.
Constructors¶
OEColor()
The default constructor initializes the color object to white (R=255, B=255, G=255, A=255).
OEColor(const OEColor &rhs)
Copy constructor.
OEColor(unsigned int packed)
Create a color object from a packed 32-bit integer. Each 8-bit byte of the integer is assumed to contain a different component of the color.
OEColor(const std::string &text)
Create a color object that is initialized with an RGB or an RGBA
hexadecimal string. The format of an RGB string is #RRGGBB
and format of an RGBA string is #RRGGBBAA
, where R,G,B and A
are hexadecimal numbers. For example, the string #FF0000
represents the color red.
OEColor(const unsigned char *rgba)
Create a color object from an array of unsigned char
. The
array is assumed to contain at least 4 bytes, where each byte
corresponds to RGBA components respectively.
OEColor(unsigned int red, unsigned int green, unsigned int blue)
Create a color object with a specified red, green, and
blue color components, the alpha component is set to
255
. Each argument should be in the range of 0
to
255
.
OEColor(unsigned int red, unsigned int green, unsigned int blue, unsigned int alpha)
Create a color object with a specified red, green, blue, and alpha components.
operator!=¶
bool operator!=(const OEColor &rhs) const
Determines whether two OEColor objects are different. Two OEColor objects are considered different, if any of their color components (red, green, blue, or alpha) are different.
operator==¶
bool operator==(const OEColor &rhs) const
Determines whether two OEColor objects are equal. Two OEColor objects are considered equivalent if all of their color components (red, green, blue, and alpha) are identical.
CreateCopy¶
OEColor *CreateCopy() const
Deep copy constructor that returns a pointer to a copy of the object. The memory for the returned OEColor object is dynamically allocated and owned by the caller.
GetR¶
unsigned int GetR() const
Returns the red component of the OEColor
object in the range of [0, 255]
.
See also
OEColor.SetR
method
GetG¶
unsigned int GetG() const
Returns the green component of the OEColor
object in the range of [0, 255]
.
See also
OEColor.SetG
method
GetB¶
unsigned int GetB() const
Returns the blue component of the OEColor
object in the range of [0, 255]
.
See also
OEColor.SetB
method
GetA¶
unsigned int GetA() const
Returns the alpha component of the OEColor
object in the range of [0, 255]
.
See also
OEColor.SetA
method
GetPackedColor¶
unsigned int GetPackedColor() const
Returns all the components of the OEColor object packed into a single 32-bit integer. Each 8-bit byte of the integer contains a different component of the color.
GetRGBA¶
const unsigned char *GetRGBA() const
Returns all the components of the OEColor object as an array of 4 bytes. The array contains 4 bytes, where each byte corresponds to RGBA components respectively. The pointer returned is owned by the OEColor object and should not be deallocated by the user.
GetText¶
std::string GetText(bool alpha=false) const
Returns the hexadecimal string representation of the
OEColor object. By default the format is
#RRGGBB
. If the alpha
argument is set to true
the
returned string will include the alpha component in the format
#RRGGBBAA
.
Set¶
OEColor &Set(unsigned int r, unsigned int g, unsigned int b)
OEColor &Set(unsigned int r, unsigned int g, unsigned int b, unsigned int a)
Sets all the components of the OEColor
object. Each argument is assumed to be in the range of 0
to
255
. Returns a reference to the OEColor
object.
SetR¶
OEColor &SetR(unsigned int r)
Sets the red component of the OEColor
object.
The given value is clamped into the range of [0, 255]
.
Returns a reference to the OEColor
object.
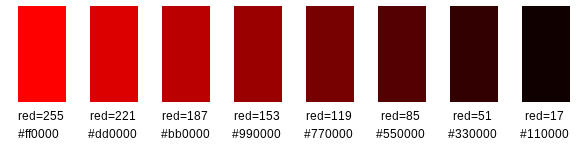
Examples of changing the red color component¶
See also
OEColor.GetR
method
SetG¶
OEColor &SetG(unsigned int g)
Sets the green component of the OEColor
object.
The given value is clamped into the range of [0, 255]
.
Returns a reference to the OEColor
object.
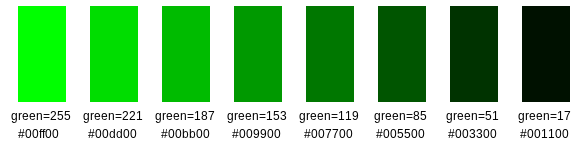
Examples of changing the green color component¶
See also
OEColor.SetG
method
SetB¶
OEColor &SetB(unsigned int b)
Sets the blue component of the OEColor
object.
The given value is clamped into the range of [0, 255]
.
Returns a reference to the OEColor
object.
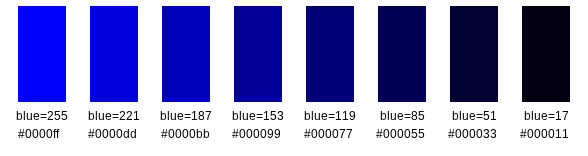
Examples of changing the blue color component¶
See also
OEColor.SetB
method
SetA¶
OEColor &SetA(unsigned int a)
Sets the alpha component of the OEColor
object.
The given value is clamped into the range of [0, 255]
.
Returns a reference to the OEColor
object.
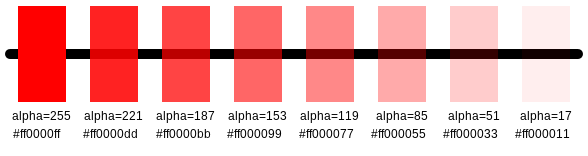
Examples of changing the alpha (transparency) color component¶
See also
OEColor.SetA
method
SetPackedColor¶
OEColor &SetPackedColor(unsigned int color)
Sets all the components of the OEColor object from a packed 32-bit integer. Each 8-bit byte of the integer is assumed to contain a different component of the color. Returns a reference to the OEColor object.
SetRGBA¶
OEColor &SetRGBA(const unsigned char *rgba)
Sets all the components of the OEColor object
from an array of unsigned char
. The array is assumed to
contain at least 4 bytes, where each byte corresponds to RGBA
components respectively. Returns a reference to the
OEColor object.
SetText¶
OEColor &SetText(const std::string &text)
Sets all the components of the OEColor object
from a RGB or RGBA hexadecimal string. The format of an RGB
string is #RRGGBB
and format of an RGBA string is
#RRGGBBAA
, where R,G,B and A are hexadecimal numbers. For
example, the string #FF0000
represents the color
red. Returns a reference to the OEColor
object.