OE2DMolDisplay¶
class OE2DMolDisplay : public OEMolDisplayBase
This class represents OE2DMolDisplay that stores the depiction information (such as atom coordinates, molecule scaling, representation styles etc.) of a molecule. See Figure: OEDepict TK molecule display class hierarchy.
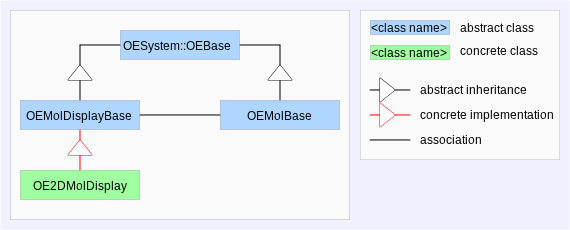
OEDepict TK molecule display class hierarchy¶
See also
OE2DMolDisplayOptions class
OE2DAtomDisplay class
OE2DBondDisplay class
The following methods are publicly inherited from OEMolDisplayBase:
Constructors¶
OE2DMolDisplay(const OEChem::OEMolBase &mol)
Initializes an OE2DMolDisplay object using default display options.
- mol
The molecule for which display information is stored in the OE2DMolDisplay object.
See also
OE2DMolDisplayOptions::Constructors
method for the list of default display optionsOE2DMolDisplay::IsValid
method
OE2DMolDisplay(const OEChem::OEMolBase &mol, const OE2DMolDisplayOptions &opts)
Initializes an OE2DMolDisplay object using the given display options.
- mol
The molecule for which display information is stored in the OE2DMolDisplay object.
- opts
The OE2DMolDisplayOptions object that stores properties that determine the styles of the molecule depiction.
Warning
An OE2DMolDisplay object does not make a copy of the OEMolBase object from which it is initialized but only stores its pointer. Therefore, the user responsibility is to not let the molecule go out of scope, before the corresponding OE2DMolDisplay object.
Changing the OEMolBase object from which OE2DMolDisplay object is initialized has no effect on the display itself after initialization. However, the OE2DMolDisplay object become invalid if the molecule graph is modified (atoms or bonds added or deleted).
Note
A warning is thrown when an OE2DMolDisplay object is initialized with a molecule with 3D coordinates.
An OE2DMolDisplay object is considered invalid and an error is thrown when it is initialized:
with an empty molecule (i.e. molecule with no atoms)
with a molecule that has neither 2D nor 3D coordinates
See also:
OE2DMolDisplay::IsValid
method
OE2DMolDisplay(const OE2DMolDisplay &rhs)
Copy constructor.
CreateCopy¶
OESystem::OEBase *CreateCopy() const
Deep copy constructor that returns a copy of the object. The memory for the returned OE2DMolDisplay object is dynamically allocated and owned by the caller.
GetAtomDisplay¶
OE2DAtomDisplay *GetAtomDisplay(const OEChem::OEAtomBase *atom)
Returns the pointer of the OE2DAtomDisplay object that stores the depiction information of the given atom.
const OE2DAtomDisplay *GetAtomDisplay(const OEChem::OEAtomBase *atom) const
Returns the const pointer of the OE2DAtomDisplay object that stores the depiction information of the given atom.
Note
The OE2DMolDisplay::GetAtomDisplay
methods
returns a zero pointer if the given atom does not correspond to any
display atom stored in the OE2DMolDisplay object.
It is a good programming practice to check the returned pointer
before using it. See code snippet below.
OE2DAtomDisplay* adisp = disp.GetAtomDisplay(aptr);
if (adisp != nullptr && adisp->IsVisible())
{
// do something
}
See also
OE2DAtomDisplay class
GetAtomDisplays¶
OESystem::OEIterBase<OE2DAtomDisplay> *GetAtomDisplays() const
Returns an iterator over all OE2DAtomDisplay objects stored in the OE2DMolDisplay object.
OESystem::OEIterBase<OE2DAtomDisplay> *
GetAtomDisplays(const OESystem::OEUnaryPredicate<OEChem::OEAtomBase> &pred) const
Returns an iterator over only those OE2DAtomDisplay objects for which the corresponding atom passes the given predicate. For example, the following code snippet shows how to access the atom displays of oxygen atoms:
OEIter<OE2DAtomDisplay> ai;
for (ai = disp.GetAtomDisplays(OEHasAtomicNum(OEElemNo::O)); ai; ++ai)
{
if (ai->IsVisible())
{
// do something
}
}
See also
OE2DAtomDisplay class
Listing 4
example in the Customizing Molecule Depiction section
GetBondDisplay¶
OE2DBondDisplay *GetBondDisplay(const OEChem::OEBondBase *bond)
Returns the pointer of the OE2DBondDisplay object, that stores the depiction information of the given bond.
const OE2DBondDisplay *GetBondDisplay(const OEChem::OEBondBase *bond) const
Returns the const pointer of the OE2DBondDisplay object, that stores the depiction information of the given bond.
See also
OE2DBondDisplay class
Note
The OE2DMolDisplay::GetBondDisplay
methods
returns a zero pointer if the given bond does not correspond to any
display bond stored in the OE2DMolDisplay object.
It is a good programming practice to check the returned pointer
before using it. See code snippet below.
OE2DBondDisplay* bdisp = disp.GetBondDisplay(bptr);
if (bdisp != nullptr && bdisp->IsVisible())
{
// do something
}
See also
OE2DBondDisplay class
GetBondDisplays¶
OESystem::OEIterBase<OE2DBondDisplay> *GetBondDisplays() const
Returns an iterator over all OE2DBondDisplay objects stored in the OE2DMolDisplay object.
OESystem::OEIterBase<OE2DBondDisplay> *
GetBondDisplays(const OESystem::OEUnaryPredicate<OEChem::OEBondBase> &pred) const
See also
OE2DBondDisplay class
Returns an iterator over only those OE2DBondDisplay objects for which the corresponding bond passes the given predicate. For example, the following code snippet shows how to access the bond displays of aromatic bonds:
OEIter<OE2DBondDisplay> bi;
for (bi = disp.GetBondDisplays(OEIsAromaticBond()); bi; ++bi)
{
if (bi->IsVisible())
{
// do something
}
}
See also
OE2DBondDisplay class
Listing 4
example in the Customizing Molecule Depiction section
GetDataType¶
const void *GetDataType() const
This function is used to perform run-time type identification.
See also
OEBase::GetDataType
method in the OEChem TK manual
GetHeight¶
double GetHeight() const
Returns the height of the displayed molecule.
See also
OE2DMolDisplay::GetScale
methodOE2DMolDisplay::GetWidth
method
GetLayer¶
OEImage &GetLayer(unsigned int position, unsigned int idx=0)
const OEImage &GetLayer(unsigned int position, unsigned int idx=0) const
Provides access to the layers of the OE2DMolDisplay
object.
Currently, an OE2DMolDisplay object has two layers
(one OELayerPosition::Above
and
one OELayerPosition::Below
) that allows
drawing of objects over or underneath the molecular structure, respectively.
- position
This value has to be from the
OELayerPosition
namespace.- idx
This parameter is currently not used.
See also
OEImage class
OELayerPosition
namespace
GetOptions¶
const OE2DMolDisplayOptions &GetOptions() const
Returns the options used to initialized the OE2DMolDisplay object.
See also
OE2DMolDisplayOptions class
GetScale¶
double GetScale() const
Returns the scaling factor of the displayed molecule.
See also
OE2DMolDisplay::GetHeight
methodOE2DMolDisplay::GetWidth
method
GetWidth¶
double GetWidth() const
Returns the width of the displayed molecule.
See also
OE2DMolDisplay::GetHeight
methodOE2DMolDisplay::GetScale
method
IsDataType¶
bool IsDataType(const void *) const
Returns whether type is the same as the instance this method is called on.
See also
OEBase::IsDataType
method in the OEChem TK manual
IsValid¶
bool IsValid() const
Returns whether the OE2DMolDisplay object was initialized successfully. If initialization was attempted with an empty molecule or a molecule with no coordinates (neither 2D nor 3D), then this method returns false. An OE2DMolDisplay object becomes invalid if the molecule, from which it was initialized, is modified. For example, atom or bonds added to or deleted from the molecule.
Hint
It is highly recommend to check whether a molecule display is valid after initialization.