Depicting Tripos Atom Types¶
Problem¶
You want to depict the Tripos atom types on your molecule. See example in Figure 1.
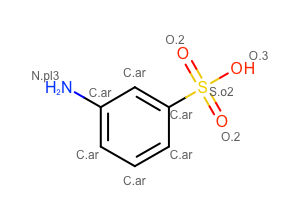
Figure 1. Example of depicting Tripos atom types
Ingredients¶
|
Difficulty Level¶

Solution¶
The OEDepict TK allows you to display any atom property as a string next to the atomic label on your molecular diagram. First you have to create a class derived from the OEDisplayAtomPropBase abstract base class of which __call__ method returns the type string of an atom.
1 2 3 4 5 6 7 8 9 10 | class TriposTypeLabel(oedepict.OEDisplayAtomPropBase):
def __init__(self):
oedepict.OEDisplayAtomPropBase.__init__(self)
def __call__(self, atom):
return atom.GetType()
def CreateCopy(self):
copy = TriposTypeLabel()
return copy.__disown__()
|
Before depicting the molecule, you have to call the OETriposAtomTypeNames function that sets the atom name property of each atom to be the Tripos type (see line 3 in the code below).
Then the TriposTypeLabel class, defined above, can be used to customize the molecule depiction. When a OE2DMolDisplay object is constructed to depict a molecule, it takes an OE2DMolDisplayOptions object that stores all the options that determine how a molecule is depicted. By default, no atom property label is rendered next to the atoms. This default behavior can be overwritten by calling the OE2DMolDisplayOptions.SetAtomPropertyFunctor method (see line 6 in the code below).
When you render the molecule the Tripos atom type label will be depicted next to the atom. See example in Figure 1.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | def DepictMoleculeWithTriposAtomTypes(image, mol):
oechem.OETriposAtomTypeNames(mol)
opts = oedepict.OE2DMolDisplayOptions(image.GetWidth(), image.GetHeight(), oedepict.OEScale_AutoScale)
opts.SetAtomPropertyFunctor(TriposTypeLabel())
disp = oedepict.OE2DMolDisplay(mol, opts)
oedepict.OERenderMolecule(image, disp)
#############################################################################
# INTERFACE
#############################################################################
|
Discussion¶
Similarly, you can also display bond property information on your molecule image. You can find examples in the Displaying Bond Properties section of the OEDepict TK manual.
See also in OEDepict TK manual¶
Theory
- Molecule Depiction chapter
API
- OE2DMolDisplay class
- OE2DMolDisplayOptions class
- OEDisplayAtomPropBase abstract base class
- OERenderMolecule function