OESubSearchScreen¶
Attention
This API is currently available in C++ and Python.
class OESubSearchScreen : public OESystem::OEBitVector
OESubSearchScreen class represents substructure search screens that encode local and global features of query and target molecules. These screens can be used to accelerate the substructure search since target molecules that clearly can not be matched to the query can be rapidly eliminated. Only molecules that pass the screening phase are subjected to the more expensive atom-by-atom validation when using the search methods of the OESubSearchDatabase class. An OESubSearchScreen objects are typed bit-vectors (OEBitVector). The type determines what kind of queries in mind the screen was designed for (SMARTS, MDL query, or molecule query).
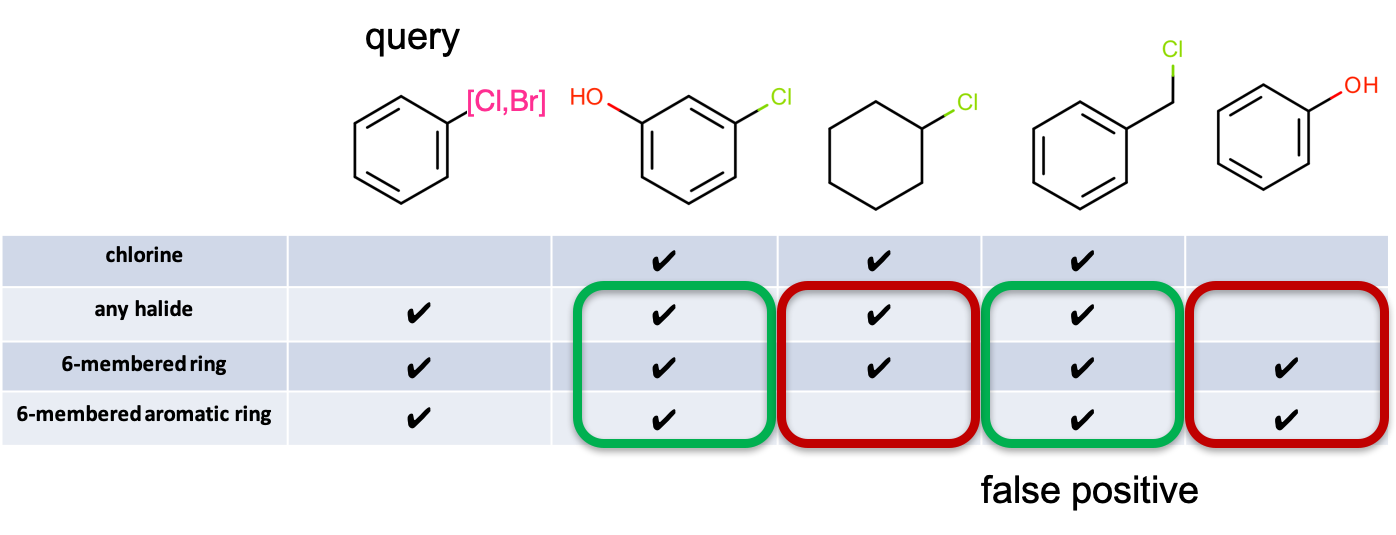
Example of using substructure search screens to eliminate target molecules¶
See also
OESubSearchScreenType
namespaceOEMakeSubSearchQueryScreen
andOEMakeSubSearchTargetScreen
functions
Constructors¶
OESubSearchScreen()
The default constructor creates an uninitialized
OESubSearchScreen object. The object can be initialized by
calling either the OEMakeSubSearchQueryScreen
function
for query molecules or the OEMakeSubSearchTargetScreen
function
for target molecules.
Examples:
The following code snippet shows how to generate substructure screens:
OEGraphMol mol;
OESmilesToMol(mol, "c1ccccc1");
OESubSearchScreen screenA;
OEMakeSubSearchTargetScreen(screenA, mol, OESubSearchScreenType::Molecule);
OESubSearchScreen screenB;
OEMakeSubSearchTargetScreen(screenB, mol, screenA.GetScreenType());
The following code snippet shows how to store and retrieve screens as generic data:
const char* tag = "SCREEN_DATA";
OEGraphMol mol;
OESmilesToMol(mol, "c1ccccc1");
OESubSearchScreen screen;
OEMakeSubSearchTargetScreen(screen, mol, OESubSearchScreenType::Molecule);
mol.SetData<OESubSearchScreen>(tag, screen);
OESubSearchScreen s = mol.GetData<OESubSearchScreen>(tag);
const OESubSearchScreenTypeBase* stype = s.GetScreenType();
cout << "molecule has `" << stype->GetName() << "` with `" << tag << "` identifier" << endl;
OESubSearchScreen(const OESubSearchScreen &)=default
Copy constructor.
GetScreenType¶
const OESubSearchScreenTypeBase *GetScreenType() const
Returns the type of the screen (OESubSearchScreenTypeBase).