Basic Drawing Primitives¶
The following example introduces how an image can be created in OEDepict TK to generate a single black line across an image:
Create an OEImage object with a specific width and height.
Specify the two end points of the line with OE2DPoint objects.
Draw the line with the
OEImage::DrawLine
method using theOEDepict::OEBlackPen
to specify the graphical properties of the line.Write the image into a file by calling the
OEWriteImage
function.The OEImage class is implemented as a container of drawing commands. When a method such as
OEImage::DrawLine
is called, rather than immediately drawing the line specified by the parameters, a line drawing operation is created in the OEImage class for later execution.
Listing 1: Example of line drawing
int main()
{
const auto width = 100u;
const auto height = 100u;
// Create image
OEImage image(width, height);
// Draw line with default pen
image.DrawLine(OE2DPoint(10.0, 10.0), OE2DPoint(90.0, 90.0), OEBlackPen);
// Write image to SVG file
OEWriteImage("DrawLine.svg", image);
return 0;
}
The image created by Listing 1
is shown in
Figure: Example of line drawing.
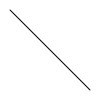
Example of line drawing¶
See also
OEPen class
OEImage class
OEWriteImage
function
Coordinate System¶
The coordinate system used in OEDepict TK has the origin (x=0.0, y=0.0) at the top left with the x-axis pointing to the right and the y-axis pointing down.
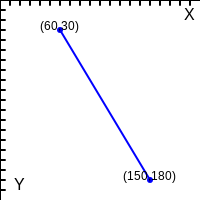
2D coordinate system (X = horizontal, Y = vertical)¶
See also
OE2DPoint class
Image File Formats¶
OEDepict TK natively supports a variety of graphical file
formats. OEWriteImage
automatically detects the
desired file format from the file name passed to the function. The
following table lists the file formats natively supported
in OEDepict TK and their associated file extensions:
Graphics File Format |
Format Type |
File Extension |
---|---|---|
PNG (Portable Network Graphics) |
|
|
SVG (Scalable Vector Graphics) |
|
|
bare SVG (with no header) |
|
|
Postscript |
|
|
Encapsulated PostScript |
|
|
PDF (Portable Document Format) |
|
The OEIsRegisteredImageFile
function can be used
to query whether a given file extension is supported by OEDepict TK.
All the supported file formats can be queried using
the OEGetSupportedImageFileExtensions
function.