OEHighlightByStick¶
class OEHighlightByStick : public OEHighlightBase
The OEHighlightByStick class allows the
customization of the stick highlighting style that is
associated with the OEHighlightStyle.Stick
constant.
See example in Figure: Example of highlighting using the
‘stick’ style.
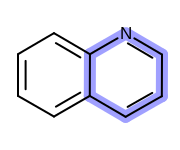
Example of highlighting using the ‘stick’ style¶
The OEHighlightByStick class stores the following properties:
Property |
Get method |
Set method |
Corresponding namespace / class / type |
---|---|---|---|
external highlight |
floating point |
||
atom label |
boolean |
||
color |
|||
stick width scale |
floating point number |
See also
OEAddHighlighting
functionOEHighlightStyle
namespaceOEHighlightByColor class
OEHighlightByCogwheel class
OEHighlightByLasso class
Highlighting chapter
Constructors¶
OEHighlightByStick(const OESystem::OEColor &color, double stickWidthScale=3.0,
bool monochrome=true, double atomExternalHighlightRatio=0.0)
Creates an OEHighlightByStick object with the specified parameters.
- color
The color used for highlighting. See also
OEHighlightByStick.SetColor
method.- stickWidthScale
The multiplier that can be used to increase or decrease the stick width of the highlighted bond(s). See also
OEHighlightByStick.SetStickWidthScale
method.- monochrome
Defines whether or not to change the color of the atom label of the highlighted atom(s). See also
OEHighlightByStick.SetAtomLabelMonochrome
method.- atomExternalHighlightRatio
A ratio that can be used to extend atom highlights to bonds not being highlighted. See also
OEHighlightByStick.SetAtomExternalHighlightRatio
method.
OEHighlightByStick(const OEHighlightByStick &rhs)
Copy constructor.
CreateCopy¶
OEHighlightBase *CreateCopy() const
Deep copy constructor that returns a copy of the object. The memory for the returned OEHighlightByStick object is dynamically allocated and owned by the caller.
GetAtomExternalHighlightRatio¶
double GetAtomExternalHighlightRatio() const
Returns the ratio of the external highlight of the atoms.
See also
GetAtomLabelMonochrome¶
bool GetAtomLabelMonochrome() const
Returns whether or not the color of the atom label of the highlighted atom(s) is changed.
See also
GetColor¶
const OESystem::OEColor &GetColor() const
Returns the color of the highlighting.
See also
OEHighlightByStick.SetColor
methodOEColor class
GetStickWidthScale¶
double GetStickWidthScale() const
Returns the multiplier that can be used to increase or decrease the stick width of the highlighted bond(s).
See also
SetAtomExternalHighlightRatio¶
void SetAtomExternalHighlightRatio(bool ratio)
Sets the ratio of the external highlight of the atoms i.e. atom highlights are extended to bonds not being highlighted.
- ratio
This value has to be in the range of
[0.0, 0.5]
.
Example (Figure: Example of using the SetAtomExternalHighlightRatio method)
OEHighlightByStick highlight = new OEHighlightByStick(oechem.getOELightOrange());
highlight.SetAtomExternalHighlightRatio(0.33);
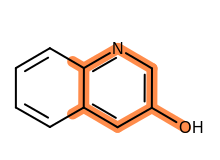
Example of using the SetAtomExternalHighlightRatio method¶
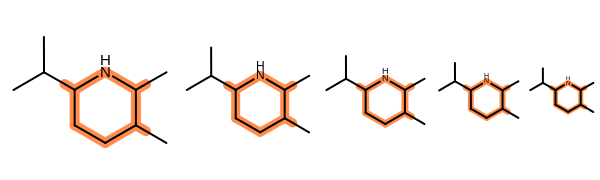
Example of scaling the ‘Stick’ style highlighting along with the molecule¶
See also
OEHighlightByStick.GetColor
method
SetAtomLabelMonochrome¶
void SetAtomLabelMonochrome(bool monochrome)
Sets whether or not to change the color of the atom label of the highlighted atom(s).
Example (Figure: Example of using the SetAtomLabelMonochrome method)
OEHighlightByStick highlight = new OEHighlightByStick(oechem.getOELightOrange());
highlight.SetAtomLabelMonochrome(false);
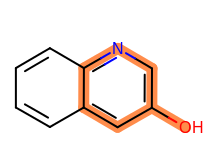
Example of using the SetAtomLabelMonochrome method¶
See also
SetColor¶
void SetColor(const OESystem::OEColor &color)
Sets the color of the highlighting.
Example (Figure: Example of using the SetColor method)
highlight.SetColor(oechem.getOELightOrange());
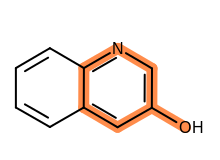
Example of using the SetColor method¶
See also
OEHighlightByStick.GetColor
methodOEColor class
SetStickWidthScale¶
void SetStickWidthScale(double stickWidthScale)
Sets the multiplier that can be used to increase or decrease the stick width of the highlighted bond(s).
Example (Figure: Example of using the SetStickWidthScale method)
OEHighlightByStick highlight = new OEHighlightByStick(oechem.getOELightOrange());
highlight.SetStickWidthScale(5.0);
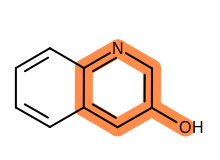
Example of using the SetStickWidthScale method¶
See also