Highlighting¶
Atoms and bonds of a molecule can be highlighted by using the
OEAddHighlighting
functions.
OEDepict TK provides four built-in highlighting styles:
- color
This style is associated with the
OEHighlightStyle.Color
constant. See Figure: Example of highlighting using the ‘color’ style.Example of highlighting using the ‘color’ style¶
- stick
This style is associated with the
OEHighlightStyle.Stick
constant. See Figure: Example of highlighting using the ‘stick’ style.Example of highlighting using the ‘stick’ style¶
- ball and stick
This style is associated with the
OEHighlightStyle.BallAndStick
constant. See Figure: Example of highlighting using the ‘ball and stick’ style.Example of highlighting using the ‘ball and stick’ style¶
- cogwheel
This style is associated with the
OEHighlightStyle.Cogwheel
constant. See Figure: Example of highlighting using the ‘cogwheel’ style.Example of highlighting using the ‘cogwheel’ style¶
- lasso
This style is associated with the
OEHighlightStyle.Lasso
constant. See Figure: Example of highlighting using the ‘lasso’ style.Example of highlighting using the ‘lasso’ style¶
Using Highlighting Styles¶
The following example (Listing 1
) shows how
to use the built-in highlighting styles.
The OEAddHighlighting
function takes an
OEMatchBase object and highlights each of its target
atoms and bonds using the given style.
In this case, the two pyridine rings in the target structure are
highlighted by red color using the stick style.
The image created by Listing 1
is shown in
Figure: Example of using ‘stick’ highlighting style.
Listing 1: Example of using highlighting style
public class HighlightSimple {
public static void main(String argv[]) {
OEGraphMol mol = new OEGraphMol();
oechem.OESmilesToMol(mol, "c1cncc2c1cc3c(cncc3)c2");
oedepict.OEPrepareDepiction(mol);
OESubSearch ss = new OESubSearch("c1ncccc1");
OE2DMolDisplay disp = new OE2DMolDisplay(mol);
boolean unique = true;
for (OEMatchBase match : ss.Match(mol, unique)) {
oedepict.OEAddHighlighting(disp, oechem.getOERed(), OEHighlightStyle.Stick, match);
}
oedepict.OERenderMolecule("HighlightSimple.svg", disp);
}
}
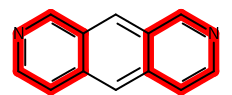
Example of using ‘stick’ highlighting style¶
See also
OEHighlightStyle
namespaceOEAddHighlighting
function
Using Highlighting Classes¶
OEDepict TK also provides the highlighting classes that correspond to the highlighting styles. Each highlighting style can be customized by using the corresponding class:
The next example (Listing 2
) shows how
the stick style can be customized by using the corresponding
OEHighlightByStick class.
The image created by Listing 2
is shown in
Figure: Example of customizing the ‘stick’ highlighting style.
Listing 2: Example of using highlighting class
public class HighlightStyle {
public static void main(String argv[]) {
OEGraphMol mol = new OEGraphMol();
oechem.OESmilesToMol(mol, "c1cncc2c1cc3c(cncc3)c2");
oedepict.OEPrepareDepiction(mol);
OESubSearch ss = new OESubSearch("c1ncccc1");
OE2DMolDisplayOptions opts = new OE2DMolDisplayOptions(240.0, 100.0, OEScale.AutoScale);
opts.SetMargins(10.0);
OE2DMolDisplay disp = new OE2DMolDisplay(mol, opts);
double stickWidthScale = 6.0;
boolean monochrome = true;
OEHighlightByStick highlight = new OEHighlightByStick(oechem.getOERed(),
stickWidthScale,
!monochrome);
boolean unique = true;
for (OEMatchBase match : ss.Match(mol, unique)) {
oedepict.OEAddHighlighting(disp, highlight, match);
}
oedepict.OERenderMolecule("HighlightStyle.svg", disp);
}
}
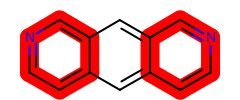
Example of customizing the ‘stick’ highlighting style¶
See also
OEAddHighlighting
function
Highlighting Overlapped Patterns¶
If more than one part of a molecule is highlighted, these highlights can overlap resulting in loss of information. For example, highlighting the matches of the c1cc[c,n]cc1 SMARTS pattern will produce the following image.
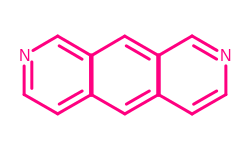
Example of highlighting multiple matches in the same image¶
There are two ways how this can be avoided and correctly
highlight overlapping parts of a molecule.
The first example demonstrates how to depict multiple matches in
different image cells one by one.
The image created by Listing 3
is shown in
Figure: Example of highlighting multiple matches one by one.
Listing 3: Example of highlighting multiple matches one by one
public class HighlightMulti {
public static void main(String argv[]) {
OEGraphMol mol = new OEGraphMol();
oechem.OESmilesToMol(mol, "c1cncc2c1cc3c(cncc3)c2");
oedepict.OEPrepareDepiction(mol);
OESubSearch ss = new OESubSearch("c1cc[c,n]cc1");
double width = 350;
double height = 250;
OEImage image = new OEImage(width, height);
int rows = 2;
int cols = 2;
OEImageGrid grid = new OEImageGrid(image, rows, cols);
OE2DMolDisplayOptions opts = new OE2DMolDisplayOptions(grid.GetCellWidth(),
grid.GetCellHeight(),
OEScale.AutoScale);
boolean unique = true;
OEImageBaseIter celliter = grid.GetCells();
for (OEMatchBase match : ss.Match(mol, unique)) {
OE2DMolDisplay disp = new OE2DMolDisplay(mol, opts);
oedepict.OEAddHighlighting(disp, oechem.getOEPink(), OEHighlightStyle.Color, match);
oedepict.OERenderMolecule(celliter.Target(), disp);
celliter.Increment();
}
oedepict.OEWriteImage("HighlightMulti.svg", image);
}
}
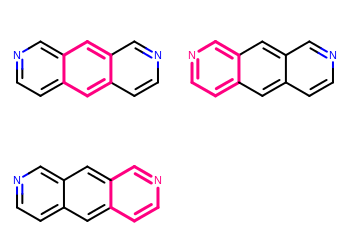
Example of highlighting multiple matches one by one¶
See also
OEImageGrid class
Depicting Molecules in a Grid section
The second example (Listing 4
) illustrates how
to highlight overlapping matches at once using the
OEHighlightOverlayByBallAndStick class.
In this example, the OEAddHighlightOverlay
function takes
all matches being highlighted and colors the overlapped atoms and bonds
using the colors by turn.
The colors used for highlighting are determined when the
OEHighlightOverlayByBallAndStick object is constructed.
The image created by Listing 4
is shown in
Figure: Example of highlighting overlapping matches at once.
Listing 4: Example of highlighting multiple matches simultaneously
public class HighlightOverlay {
public static void main(String argv[]) {
OEGraphMol mol = new OEGraphMol();
oechem.OESmilesToMol(mol, "c1cncc2c1cc3c(cncc3)c2");
oedepict.OEPrepareDepiction(mol);
OESubSearch subs = new OESubSearch("c1cc[c,n]cc1");
OE2DMolDisplayOptions opts = new OE2DMolDisplayOptions(240.0, 100.0, OEScale.AutoScale);
opts.SetMargins(10.0);
OE2DMolDisplay disp = new OE2DMolDisplay(mol, opts);
OEHighlightOverlayByBallAndStick highlight =
new OEHighlightOverlayByBallAndStick(oechem.OEGetContrastColors());
boolean unique = true;
oedepict.OEAddHighlightOverlay(disp, highlight, subs.Match(mol, unique));
oedepict.OERenderMolecule("HighlightOverlay.png", disp);
}
}
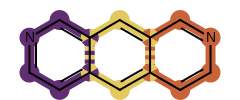
Example of highlighting overlapping matches at once¶
See also
OEHighlightOverlayBase base class
OEHighlightOverlayStyle
namespaceOEGetColors
,OEGetContrastColors
,OEGetDeepColors
,OEGetLightColors
andOEGetVividColors
functions