OEFont¶
class OEFont
The OEFont class encapsulates properties that determine the display style of texts.
Currently the OEFont class stores the following properties:
Property |
Get method |
Set method |
Corresponding namespace/class/type |
---|---|---|---|
alignment |
|||
color |
|||
font family |
|||
font size |
None |
||
font style |
|||
hyperlink |
string |
||
rotation angle |
floating point number in range of \([0.0^{\circ}, 360.0^{\circ}]\) |
See also
OEImageBase::DrawText
method
Constructors¶
OEFont()
Default constructor that initializes an OEFont object with the following properties: See example in Figure: Example of drawing text with default OEFont object.
Property |
Default value |
---|---|
alignment |
|
color |
|
font family |
|
font size |
12 |
hyperlink |
empty string (i.e. no hyperlink) |
font style |
|
rotation angles |
0.0 (i.e. no rotation) |
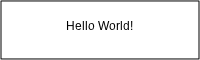
Example of drawing text with default OEFont object¶
OEFont(const OESystem::OEColor &color)
Constructor that initializes an OEFont object with the default parameters and the given OEColor.
OEFont(unsigned int family, unsigned int style, unsigned int size,
unsigned int align, const OESystem::OEColor &color,
double rotangle = 0.0)
Constructor that initializes an OEFont object with the given parameters.
- family
The family of the font. This value has to be from the
OEFontFamily
namespace. See alsoOEFont::SetFamily
method.- style
The style of the font. This value has to be from the
OEFontStyle
namespace. See alsoOEFont::SetSize
method.- size
The size of the font. See also
OEFont::SetSize
method.- align
The alignment of the text. This value has to be from the
OEAlignment
namespace. See alsoOEFont::SetAlignment
method.- color
The color of the font. See also
OEFont::SetColor
method.- rotangle
The rotation angle of the text. The default value is 0.0 which means no rotation is applied i.e. the text using the OEFont object is rendered horizontally. See also
OEFont::SetRotationAngle
method.
OEFont(const OEFont &rhs)
Copy constructors.
operator!=¶
bool operator!=(const OEFont &rhs) const
Determines whether two OEFont objects are different. Two OEFont objects are considered different if any of their properties (such as color, size, etc.) are different.
operator==¶
bool operator==(const OEFont &rhs) const
Determines whether two OEFont objects are equal. Two OEFont objects are considered equivalent only if all of their properties (such as color, size, etc.) are identical.
GetAlignment¶
unsigned int GetAlignment() const
Returns the alignment property of the OEFont
object.
The returned value is taken from the OEAlignment
namespace.
See also
OEFont::SetAlignment
methodOEAlignment
namespace
GetColor¶
const OESystem::OEColor &GetColor() const
Returns the color of the OEFont object.
See also
OEFont::SetColor
methodOEColor class
GetFamily¶
unsigned int GetFamily() const
Returns the font family property of the OEFont
object.
The returned value is taken from the OEFontFamily
namespace.
See also
OEFont::SetFamily
methodOEFontFamily
namespace
GetHyperlink¶
const std::string& GetHyperlink() const
Returns the URI string associated with the OEFont object.
See also
OEFont::HasHyperlink
methodOEFont::SetHyperlink
method
GetRotationAngle¶
double GetRotationAngle() const
Returns the angle of the rotation that is used to render a text when using the OEFont object.
See also
OEFont::SetRotationAngle
method
GetSize¶
unsigned int GetSize() const
Returns the size of the OEFont object.
See also
OEFont::SetSize
method
GetStyle¶
unsigned int GetStyle() const
Returns the font style property of the OEFont
object.
The returned value is taken from the OEFontStyle
namespace.
See also
OEFont::SetStyle
methodOEFontStyle
namespace
HasHyperlink¶
bool HasHyperlink() const
Returns whether an URI string is associated with the OEFont object.
See also
OEFont::GetHyperlink
methodOEFont::SetHyperlink
method
SetAlignment¶
void SetAlignment(unsigned int align)
Sets the alignment property of the OEFont object.
- align
This value has to be from the
OEAlignment
namespace.
Example (Figure: Example of using the SetAlignment method)
OEFont font;
font.SetAlignment(OEAlignment::Right);
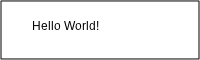
Example of using the SetAlignment method¶
See also
OEFont::GetAlignment
methodOEAlignment
namespace
SetColor¶
void SetColor(const OESystem::OEColor &color)
Sets the color of the OEFont object.
Example (Figure: Example of using the SetColor method)
OEFont font;
font.SetColor(OERed);
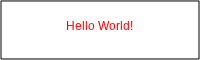
Example of using the SetColor method¶
See also
OEFont::GetColor
methodOEColor class
SetFamily¶
void SetFamily(unsigned int family)
Sets the font family property of the OEFont object.
- family
This value has to be from the
OEFontFamily
namespace.
Example (Figure: Example of using the SetFamily method)
OEFont font;
font.SetFamily(OEFontFamily::Times);
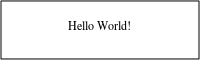
Example of using the SetFamily method¶
See also
OEFont::GetFamily
methodOEFontFamily
namespace
SetHyperlink¶
void SetHyperlink(const string& uri)
Associates URI string with the OEFont object.
- uri
After associating a valid URI with a OEFont object, texts drawn with the given font become “click-able” and by clicking on them will send you to the given address.
Example (Figure: Example of using the SetHyperlink method)
OEGraphMol mol;
OESmilesToMol(mol, "OC(=O)c1ccccc1");
OEPrepareDepiction(mol);
const double width = 150.0;
const double height = 150.0;
OEImage image(width, height);
OE2DMolDisplayOptions opts(width, height, OEScale::AutoScale);
opts.SetTitleLocation(OETitleLocation::Bottom);
// associate font with URI
OEFont font(OEFontFamily::Default, OEFontStyle::Default, 10u, OEAlignment::Center, OEBlue);
font.SetHyperlink("http://www.eyesopen.com/oedepict-tk");
opts.SetTitleFont(font);
mol.SetTitle("Generated by OEDepict");
OE2DMolDisplay disp(mol, opts);
OERenderMolecule(image, disp);
OEWriteImage("DepictMolWithHyperlink.pdf", image);
OEWriteImage("DepictMolWithHyperlink.svg", image);
Example of using the SetHyperlink method¶
(Clicking on the blue text at the bottom send you to the OEDepict TK web page)
Note
Associating texts with hyperlinks is only available for .pdf
and .svg
image formats.
Warning
OEDepict TK does not check whether the given string is a valid URI.
It is recommended to always keep the URI scheme
prefix of the URI string for the sake
of compatibility.
For example, some application can interpret the www.eyesopen.com
string as a valid
URI,
others only work when using http://www.eyesopen.com
.
See also
OEFont::GetHyperlink
methodOEFont::GetHyperlink
method
SetRotationAngle¶
void SetRotationAngle(double angle)
Sets the rotation angle of the OEFont object.
- angle
The rotation angle of the text (in degrees). The value of the angle has to be in a range of \([0.0^{\circ}, 360.0^{\circ}]\).
Example (Figure: Example of using the SetRotationAngle method)
OEFont font;
font.SetRotationAngle(180.0);
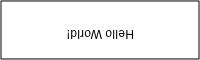
Example of using the SetRotationAngle method¶
Note
The angles are interpreted in an anti-clockwise direction. The \(0.0^{\circ}\) and \(360.0^{\circ}\) degrees are at the 3 o’clock position i.e. the text is rendered horizontally. While the \(180.0^{\circ}\) degrees corresponds to rendering the text upside down. See examples in Figure: Example of drawing texts with various rotation angle.
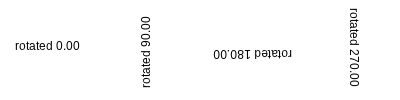
Example of drawing texts with various rotation angles¶
See also
OEFont::GetRotationAngle
method
SetSize¶
void SetSize(unsigned int size)
Sets the size of the OEFont object.
Example (Figure: Example of using the SetSize method)
OEFont font;
font.SetSize(30u);
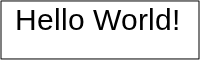
Example of using the SetSize method¶
See also
OEFont::GetSize
method
SetStyle¶
void SetStyle(unsigned int style)
Sets the font style property (such as bold, italic etc.) of the OEFont object.
- style
This value has to be from the
OEFontStyle
namespace.
Example (Figure: Example of using the SetStyle method)
OEFont font;
font.SetStyle(OEFontStyle::Bold|OEFontStyle::Italic);
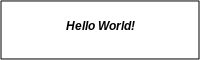
Example of using the SetStyle method¶
See also
OEFont::GetStyle
methodOEFontStyle
namespace