OEAddLabel¶
Adds label to an image or molecule display.
Link |
Description |
---|---|
adding label to an image |
|
adding label to atoms of a match |
|
adding label to set of atoms |
|
adding label to atoms specified by a predicate |
Note
When adding label associated with atoms it is recommended to use the
OEAddLabel
along with the OEAddHighlighting
function for visual clarity.
void OEAddLabel(OEImageBase& image, const OE2DPoint& center,
const OEHighlightLabel& label)
- image
The image in which the label is drawn.
- center
The center of the label on the image.
- label
The OEHighlightLabel object that stores properties that determine the styles of the label along with the text of the label itself.
Example:
void OEAddLabel_OEImage(OEImageBase& image)
{
OEHighlightLabel label("Hello!");
OEAddLabel(image, OE2DPoint(50, 50), label);
label.SetBoundingBoxPen(OETransparentPen);
OEAddLabel(image, OE2DPoint(100, 50), label);
label.SetBoundingBoxPen(OELightGreyPen);
OEAddLabel(image, OE2DPoint(150, 50), label);
}
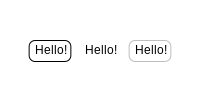
Example of adding labels to an image¶
void OEAddLabel(OE2DMolDisplay &disp,
const OEHighlightLabel &label,
const OEChem::OEMatchBase &match)
Adds label to atoms of an OEMatchBase object that can be initialized by substructure search or maximum common substructure search.
- disp
The OE2DMolDisplay object on which the label is going to be positioned.
- label
The OEHighlightLabel object that stores properties that determine the styles of the label along with the text of the label itself.
- match
The label is positioned based on the target atoms of the OEMatchBase object.
Example:
void OEAddLabel_OEMatch(OE2DMolDisplay& disp)
{
const OEMolBase* mol = disp.GetMolecule();
OESubSearch ss("a1aaaaa1");
const bool unique = true;
OEHighlightByBallAndStick highlight(OELightGreen);
for (OEIter<const OEMatchBase> mi = ss.Match(*mol, unique); mi; ++mi)
{
OEAddHighlighting(disp, highlight, mi);
OEHighlightLabel label("aromatic", OELightGreen);
OEAddLabel(disp, label, mi);
}
}
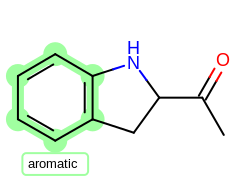
Example of adding label to 6-membered aromatic ring¶
void OEAddLabel(OE2DMolDisplay &disp,
const OEHighlightLabel &label,
const OEChem::OEAtomBondSet &abset)
Adds label to atoms stored in the OEAtomBondSet object.
- disp
The OE2DMolDisplay object on which the label is going to be positioned.
- label
The OEHighlightLabel object that stores properties that determine the styles of the label along with the text of the label itself.
- abset
The label is positioned based on the atoms stored in the OEAtomBondSet object.
Example:
void OEAddLabel_OEAtomBondSet(OE2DMolDisplay& disp)
{
const OEMolBase* mol = disp.GetMolecule();
const OEAtomBondSet ringset(mol->GetAtoms(OEAtomIsInRing()),
mol->GetBonds(OEBondIsInRing()));
OEHighlightByBallAndStick ringhighlight(OELightGreen);
OEAddHighlighting(disp, ringhighlight, ringset);
OEHighlightLabel ringlabel("ring", OELightGreen);
OEAddLabel(disp, ringlabel, ringset);
const OEAtomBondSet chainset(mol->GetAtoms(OEAtomIsInChain()),
mol->GetBonds(OEBondIsInChain()));
OEHighlightByBallAndStick chainhighlight(OEBlueTint);
OEAddHighlighting(disp, chainhighlight, chainset);
OEHighlightLabel chainlabel("chain", OEBlueTint);
OEAddLabel(disp, chainlabel, chainset);
}
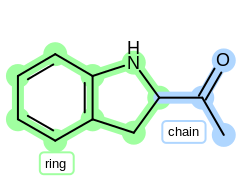
Example of adding label to ring and chain components of a molecule¶
void OEAddLabel(OE2DMolDisplay &disp,
const OEHighlightLabel &label,
const OESystem::OEUnaryPredicate<OEChem::OEAtomBase> &atompred)
Adds label to atoms for which the given atom predicate returns true.
- disp
The OE2DMolDisplay object on which the label is going to be positioned.
- label
The OEHighlightLabel object that stores properties that determine the styles of the label along with the text of the label itself.
- atompred
The label is positioned based on the atoms for which the given atom predicate returns true.
Example:
void OEAddLabel_Predicate(OE2DMolDisplay& disp)
{
OEHighlightByBallAndStick ringhighlight(OELightGreen);
OEAddHighlighting(disp, ringhighlight, OEAtomIsInRing(), OEBondIsInRing());
OEHighlightLabel ringlabel("ring", OELightGreen);
OEAddLabel(disp, ringlabel, OEAtomIsInRing());
OEHighlightByBallAndStick chainhighlight(OEBlueTint);
OEAddHighlighting(disp, chainhighlight, OEAtomIsInChain(), OEBondIsInChain());
OEHighlightLabel chainlabel("chain", OEBlueTint);
OEAddLabel(disp, chainlabel, OEAtomIsInChain());
}
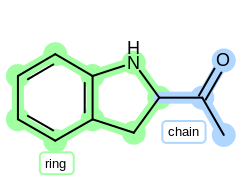
Example of adding label to ring and chain components of a molecule¶