OEImageTableOptions¶
class OEImageTableOptions
This class represents the OEImageTableOptions class that encapsulates properties that determine how an image table is depicted.
The OEImageTableOptions class stores the following properties:
Property |
Get method |
Set method |
Corresponding namespace / class / type |
---|---|---|---|
number of columns |
integer greater than 0 |
||
number of rows |
integer greater than 1 |
||
width of columns |
vector of non zero integers |
||
height of rows |
vector of non zero integers |
||
cell font size |
positive non-zero integer |
||
pen used around cells cells |
|||
table cell color |
|||
table cell font |
|||
header |
boolean |
||
header color |
|||
header font |
|||
table margins |
positive floating point number in the range of \([0.0, 25.0]\) |
||
stub column |
boolean |
||
stub column color |
|||
stub column font |
See also
Depicting Molecules in a Table section
OEImageTable class
Constructors¶
OEImageTableOptions(unsigned int rows, unsigned int cols,
unsigned int style=OEImageTableStyle::Default)
Creates an OEImageTableOptions object with the given parameters.
- rows
The total number of rows in the table including the header.
- cols
The total number of columns in the table including the stub column.
- style
This value has to be from the
OEImageTableStyle
namespace.
Note
By default, the columns and rows of a table are created with equal
widths and heights.
The relative width and height ratio of the columns and rows can be adjusted using the
OEImageTableOptions.SetColumnWidths
and
OEImageTableOptions.SetRowHeights
methods,
respectively.
Example (Example of generating a 4 x 4 image table with header and ‘MediumBlue’ style)
OEImageTableOptions tableopts = new OEImageTableOptions(4, 4, OEImageTableStyle.MediumBlue);
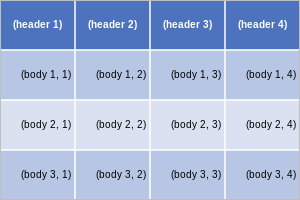
Example of generating a 4 x 4 image table with header and ‘MediumBlue’ style¶
See also
OEImageTableStyle
namespaceOEImageTableOptions.NumRows
method
OEImageTableOptions(const OEImageTableOptions &rhs)
Copy constructor.
GetCellBorderPen¶
const OEPen &GetCellBorderPen() const
Returns the pen that is used to mark the border of the cells in the table.
See also
OEPen class
GetCellColor¶
const OESystem::OEColor GetCellColor(bool even=true) const
See also
Returns the color used to highlight the ‘body’ cells in the table. A cell is considered to be part of the ‘body’ of the table if it is neither in the header nor in the stub column.
- even
This parameter determines whether to return the color of the even or odd rows in the table.
See also
OEColor class
GetCellFont¶
const OEFont GetCellFont() const
Returns the font used by the OEImageTable.DrawText
method to draw text on the ‘body’ cells.
See also
OEFont class
GetHeaderColor¶
const OESystem::OEColor GetHeaderColor() const
Returns the color used to highlight the ‘header’ cells in the table.
See also
OEColor class
GetHeaderFont¶
const OEFont GetHeaderFont() const
Returns the font used by the OEImageTable.DrawText
method to draw text on the ‘header’ cells.
See also
OEFont class
GetMargin¶
double GetMargin(unsigned int margin) const
Returns the ratio of a specific margin of the table.
- margin
This value has to be from the
OEMargin
namespace.
See also
GetStubColumnColor¶
const OESystem::OEColor GetStubColumnColor() const
Returns the color used to highlight the ‘stub column’ cells in the table.
See also
OEColor class
GetStubColumnFont¶
const OEFont GetStubColumnFont() const
Returns the font used by the OEImageTable.DrawText
method to draw text on the ‘stub column’ cells.
See also
OEFont class
HasHeader¶
bool HasHeader() const
Returns whether a table has a ‘header’ row. By default, a table is generated with a ‘header’ row.
See also
HasStubColumn¶
bool HasStubColumn() const
Returns whether the table has a ‘stub’ column. By default, a table is generated without a ‘stub’ column.
See also
NumColumns¶
unsigned int NumColumns() const
Returns the total number of columns in the table including the stub column.
NumRows¶
unsigned int NumRows() const
Returns the total number of rows in the table including the header row.
SetBaseFontSize¶
void SetBaseFontSize(unsigned int fontsize)
Controls the font size in add table cells (including cells of header, stub column and body).
See also
The other properties of the used fonts can be controlled with the following methods:
SetCellBorderPen¶
void SetCellBorderPen(const OEPen &pen)
Sets the pen that is used to mark the border of the cells in the table.
Example (Example of using the SetCellBorderPen method)
OEImageTableOptions tableopts = new OEImageTableOptions(4, 4, OEImageTableStyle.MediumBlue);
OEPen pen = new OEPen(oechem.getOEBlack(), oechem.getOEDarkBlue(), OEFill.Off, 2.0);
tableopts.SetCellBorderPen(pen);
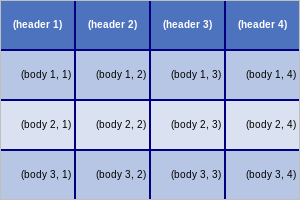
Example of using the SetCellBorderPen method¶
See also
OEPen class
SetCellColor¶
void SetCellColor(const OESystem::OEColor &color, bool even=true)
Sets the color used to highlight the ‘body’ cells in the table. A cell is considered to be part of the ‘body’ of the table if it is neither in the header nor in the stub column.
- color
The color of the cells.
- even
This parameter determines whether the color is applied to the even or to the odd rows of the table.
Example (Example of using the SetCellColor method)
OEImageTableOptions tableopts = new OEImageTableOptions(4, 4, OEImageTableStyle.MediumBlue);
boolean even = true;
tableopts.SetCellColor(oechem.getOELightGrey(), !even);
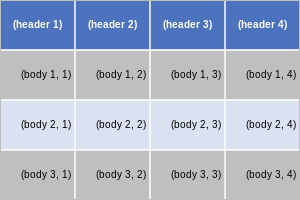
Example of using the SetCellColor method¶
See also
OEColor class
SetCellFont¶
void SetCellFont(const OEFont &font)
Sets the font used by the OEImageTable.DrawText
method to draw text on the ‘body’ cells.
A cell is considered to be part of the ‘body’ of the table if it is neither
in the header nor in the stub column.
Example (Example of using the SetCellFont method)
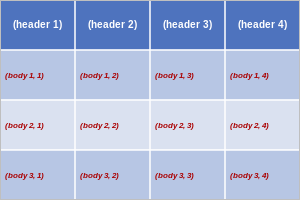
Example of using the SetCellFont method¶
See also
SetColumnWidths¶
bool SetColumnWidths(const std::vector<unsigned int> &widths)
Sets the relative widths of the columns.
- widths
The relative widths of the columns. The size of the vector has to be
OEImageTableOptions.NumColumns()
.
Example (Example of using the SetColumnWidths method)
The following example show how to adjust the width ratio of the columns.
OEImageTableOptions tableopts = new OEImageTableOptions(4, 4, OEImageTableStyle.MediumBlue);
ArrayList<Integer> widths = new ArrayList<Integer>();
widths.add(20);
widths.add(10);
widths.add(20);
widths.add(10);
tableopts.SetColumnWidths(widths);
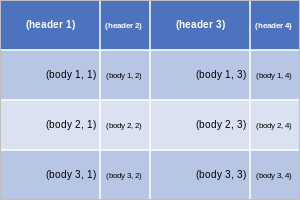
Example of using the SetColumnWidths method¶
See also
SetHeader¶
bool SetHeader(bool has)
Sets whether to have a ‘header’ row in the table.
Example (Example of using the SetHeader method)
By default, a table is generated with a header row on the top. The following example show how to remove the header.
OEImageTableOptions tableopts = new OEImageTableOptions(4, 4, OEImageTableStyle.MediumBlue);
tableopts.SetHeader(false);
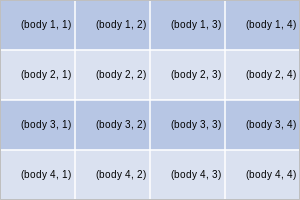
Example of using the SetHeader method¶
See also
SetHeaderColor¶
void SetHeaderColor(const OESystem::OEColor &color)
Sets the color used to highlight the ‘header’ cells in the table.
Example (Example of using the SetHeaderColor method)
OEImageTableOptions tableopts = new OEImageTableOptions(4, 4, OEImageTableStyle.MediumBlue);
tableopts.SetHeaderColor(oechem.getOELightGrey());
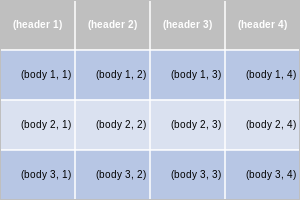
Example of using the SetHeaderColor method¶
See also
OEColor class
SetHeaderFont¶
void SetHeaderFont(const OEFont &font)
Sets the font used by the OEImageTable.DrawText
method to draw text on the ‘header’ cells.
Example (Example of using the SetHeaderFont method)
OEImageTableOptions tableopts = new OEImageTableOptions(4, 4, OEImageTableStyle.MediumBlue);
OEFont font = new OEFont(OEFontFamily.Default, OEFontStyle.Default,
12, OEAlignment.Right, oechem.getOEPinkTint());
tableopts.SetHeaderFont(font);
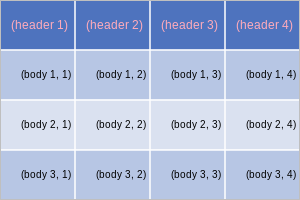
Example of using the SetHeaderFont method¶
See also
OEFont class
SetMargin¶
bool SetMargin(unsigned int marginloc, double margin)
Sets the size of a specific margin of the table.
- marginloc
This value has to be from the
OEMargin
namespace.- margin
This number is considered as a percentage of either the width or the height of image on which the table will be drawn and has to be in the range of \([0.0, 25.0]\). For example, 10.0% means, that the left and right margin are 10% of the total width of the image, and the top and bottom margins are 10% of the total height of the image.
Example (Example of using the SetMargin method)
OEImageTableOptions tableopts = new OEImageTableOptions(4, 4, OEImageTableStyle.MediumBlue);
tableopts.SetMargin(OEMargin.Left, 10.0);
tableopts.SetMargin(OEMargin.Right, 10.0);
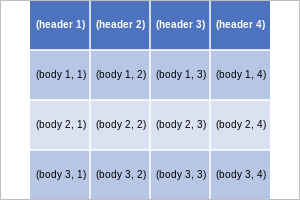
Example of using the SetMargin method¶
See also
SetMargins¶
bool SetMargins(double margin)
Sets the size of all margins of the table.
- margin
This number is considered as a percentage of either the width or the height of image on which the table will be drawn and has to be in the range of \([0.0, 25.0]\). For example, 10.0% means, that the left and right margin are 10% of the total width of the image, and the top and bottom margins are 10% of the total height of the image.
Example (Example of using the SetMargins method)
OEImageTableOptions tableopts = new OEImageTableOptions(4, 4, OEImageTableStyle.MediumBlue);
tableopts.SetMargins(10.0);
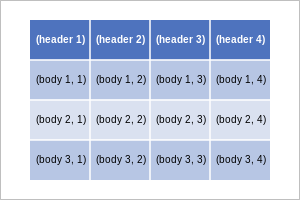
Example of using the SetMargins method¶
See also
SetRowHeights¶
bool SetRowHeights(const std::vector<unsigned int> &heights)
Sets the relative heights of the rows.
- heights
The relative heights of the rows. The size of the vector has to be
OEImageTableOptions.NumRows()
.
Example (Example of using the SetRowHeights method)
The following example show how to adjust the height ratio of the rows.
EImageTableOptions tableopts = new OEImageTableOptions(4, 4, OEImageTableStyle.MediumBlue);
rrayList<Integer> heights = new ArrayList<Integer>();
eights.add(10);
eights.add(20);
eights.add(30);
eights.add(40);
ableopts.SetRowHeights(heights);
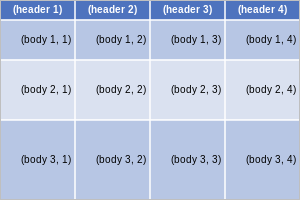
Example of using the SetRowHeights method¶
See also
SetStubColumn¶
bool SetStubColumn(bool has)
Sets whether to have a ‘stub’ column in the table.
Example (Example of using the SetStubColumn method)
By default, a table is generated without a ‘stub’ column. The following example show how to add one on the left.
OEImageTableOptions tableopts = new OEImageTableOptions(4, 4, OEImageTableStyle.MediumBlue);
tableopts.SetStubColumn(true);
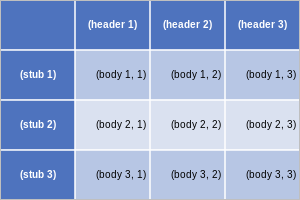
Example of using the SetStubColumn method¶
See also
SetStubColumnColor¶
void SetStubColumnColor(const OESystem::OEColor & color)
Sets the color used to highlight the ‘stub’ cells in the table.
Example (Example of using the SetStubColumnColor method)
OEImageTableOptions tableopts = new OEImageTableOptions(4, 4, OEImageTableStyle.MediumBlue);
tableopts.SetStubColumn(true);
tableopts.SetStubColumnColor(oechem.getOELightGrey());
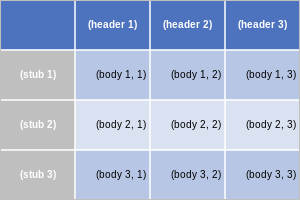
Example of using the SetStubColumnColor method¶
See also
OEColor class
SetStubColumnFont¶
void SetStubColumnFont(const OEFont &font)
Sets the font used by the OEImageTable.DrawText
method to draw text on the ‘stub column’ cells.
Example (Example of using the SetStubColumnFont method)
OEImageTableOptions tableopts = new OEImageTableOptions(4, 4, OEImageTableStyle.MediumBlue);
tableopts.SetStubColumn(true);
OEFont font = new OEFont(OEFontFamily.Default, OEFontStyle.Default,
12, OEAlignment.Right, oechem.getOEPinkTint());
tableopts.SetStubColumnFont(font);
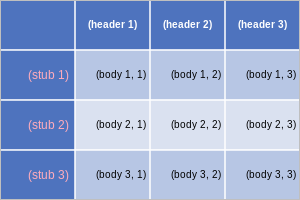
Example of using the SetStubColumnFont method¶
See also
OEFont class