Working with Szmap TK¶
The Szmap TK has a simple API, consisting of a few basic API and a couple of objects to configure the calculations (OESzmapEngine and OESzmapEngineOptions) and hold calculated results (OESzmapResults).
Preparing Protein and Ligand Molecules¶
To perform the necessary electrostatics calculations,
a protein or any other molecular context
being analyzed with SZMAP must already
have all hydrogens explicitly represented as well as have charges and radii assigned
before it can be used. This is done automatically as part of protein preparation
with Spruce TK (see OEMakeDesignUnits
).
Charges can also be assigned using Quacpac TK (see OEAssignCharges
).
Calculating Energies¶
To calculate probe energies, we initialize
a OESzmapEngine with a molecular
context, test if the point of interest is clashing,
and if not, calculate the energies. In the example below,
the energies at each ligand atom coordinate
are returned in a OESzmapResults
object, which is accessed for specific values.
Alternatively, OECalcSzmapValue
could
be used to get a single OEEnsemble
value directly.
void GetSzmapEnergies(const OEMolBase& lig, const OEMolBase& prot)
{
//run szmap at ligand coordinates in the protein context
//lig: mol defining coordinates for szmap calcs
//prot: context mol for szmap calcs (must have charges and radii)
OEThrow.Info("num\tatom\t%s\t%s\t%s\t%s\t%s",
OEGetEnsembleName(OEEnsemble::NeutralDiffDeltaG),
OEGetEnsembleName(OEEnsemble::PSolv),
OEGetEnsembleName(OEEnsemble::WSolv),
OEGetEnsembleName(OEEnsemble::VDW),
OEGetEnsembleName(OEEnsemble::OrderParam) );
float coord[3u];
OESzmapEngine sz(prot);
OESzmapResults rslt;
unsigned int i = 0u;
for (OEIter<OEAtomBase> atom = lig.GetAtoms(); atom; ++atom, ++i)
{
lig.GetCoords(atom, coord);
if (! OEIsClashing(sz, coord))
{
OECalcSzmapResults(rslt, sz, coord);
OEThrow.Info("%2d\t%s\t%.3f\t%.3f\t%.3f\t%.3f\t%.3f",
i, atom->GetName(),
rslt.GetEnsembleValue(OEEnsemble::NeutralDiffDeltaG),
rslt.GetEnsembleValue(OEEnsemble::PSolv),
rslt.GetEnsembleValue(OEEnsemble::WSolv),
rslt.GetEnsembleValue(OEEnsemble::VDW),
rslt.GetEnsembleValue(OEEnsemble::OrderParam) );
}
else
OEThrow.Info("%2d\t%s CLASH", i, atom->GetName());
}
}
The five OEEnsemble
values
in the example above are the primary ones
for understanding solvent/ligand competition.
See also
GetSzmapEnergies example
Generating Probe Orientations¶
In addition to energy calculations, the Szmap TK
can be used to generate 3D conformations of probe
molecules at calculated points, annotated
with OEComponent
energies for each conformation. In the example below,
individual atoms are also generated at calculation
points, each annotated with OEEnsemble
values.
void GenerateSzmapProbes(oemolostream& oms,
double cumulativeProb,
const OEMolBase& lig,
const OEMolBase& prot)
{
//generate multiconf probes and data-rich points at ligand coords
//oms: output mol stream for points and probes
//cumulativeProb: cumulative probability for cutoff of point set
//lig: mol defining coordinates for szmap calcs
//prot: context mol for szmap calcs (must have charges and radii)
float coord[3u];
OESzmapEngine sz(prot);
OESzmapResults rslt;
OEGraphMol points;
std::string title = "points ";
title += lig.GetTitle();
points.SetTitle(title.c_str());
OEMol probes;
unsigned int i = 0u;
for (OEIter<OEAtomBase> atom = lig.GetAtoms(); atom; ++atom, ++i)
{
lig.GetCoords(atom, coord);
if (! OEIsClashing(sz, coord))
{
OECalcSzmapResults(rslt, sz, coord);
rslt.PlaceNewAtom(points);
bool clear = false;
rslt.PlaceProbeSet(probes, cumulativeProb, clear);
}
}
OEWriteMolecule(oms, points);
OEWriteMolecule(oms, probes);
}
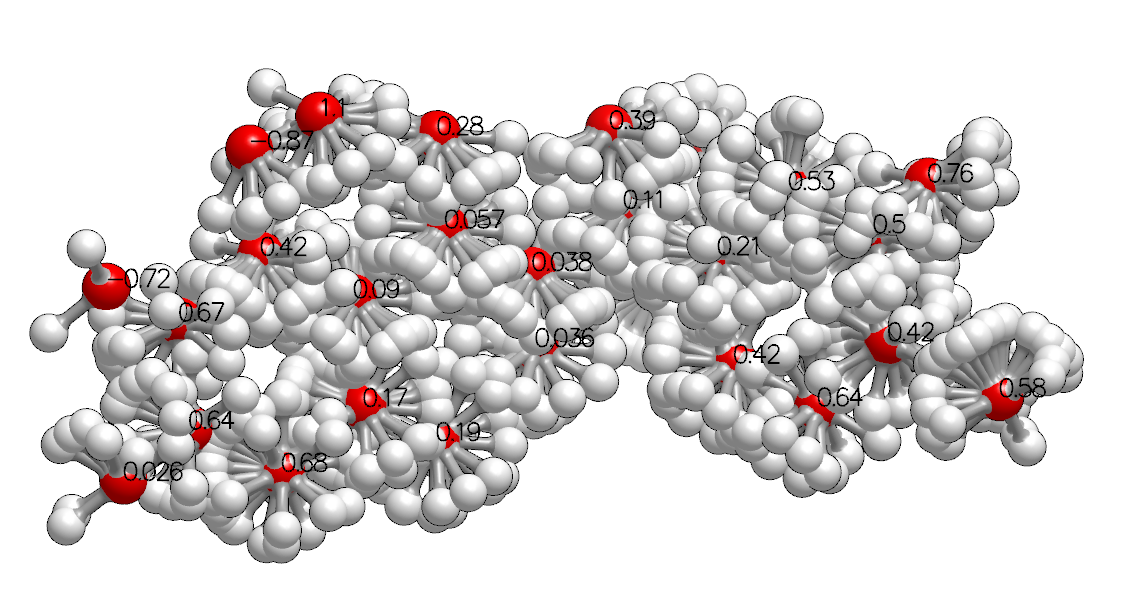
High Probability Probe Orientations and Points with Generic Data Annotation¶
See also
SzmapBestOrientations example