OE2DAtomDisplay¶
class OE2DAtomDisplay : public OEAtomDisplayBase
This class stores 2D depiction information of an atom. See Figure: OEDepict TK atom display class hierarchy.
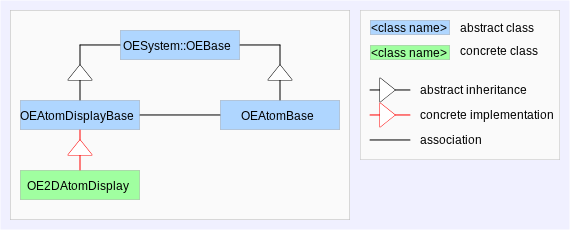
OEDepict TK atom display class hierarchy¶
The following figure (Figure: Example of the layout of an atom) illustrates how atoms are rendered in the OEDepict TK.
The atom label is positioned at the coordinates returned by the
OE2DAtomDisplay.GetCoords
method. The string that is rendered as an atom label is returned by theOE2DAtomDisplay.GetLabel
method.If the atom has a charge, then it is rendered as a super-script on the right side of the atomic label.
See also
OE2DAtomDisplay.GetCharge
method
If the atom has isotope information, then it is rendered as a super-script on the left side of the atomic label.
See also
OE2DAtomDisplay.GetMass
method
If the atom has implicit hydrogen(s), then the hydrogen label is positioned in a cardinal direction relative to the atomic label. The number of implicit hydrogens and the position can be accessed by calling the
OE2DAtomDisplay.GetHCount
and theOE2DAtomDisplay.GetHPosition
methods, respectively.See also
OEHydrogenStyle
namespaceOEHydrogenPos
namespace
All labels (isotope, atomic, charge and explicit hydrogen) has the same color that is associated with the atomic number.
See also
OEAtomColorStyle
namespaceAppendix: Element coloring (CPK) chapter
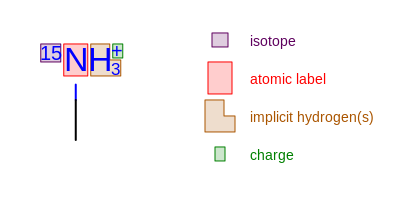
Example of the layout of an atom¶
The following methods are publicly inherited from OEAtomDisplayBase:
The OE2DAtomDisplay class stores the following properties:
Property |
Get method |
Set method |
Corresponding namespace / class / type |
---|---|---|---|
rendered formal charge |
integer |
||
position of the atom label |
OE2DPoint class |
||
hydrogen count |
unsigned int |
||
position of hydrogen label |
|
||
rendered atom label |
string |
||
font of atom label |
OEFont class |
||
rendered isotope number |
integer |
||
rendered string as property |
string |
||
font of property string |
OEFont class |
||
position of property label |
OE2DPoint class |
||
rendered radical type |
|
Constructors¶
OE2DAtomDisplay(const OEChem::OEAtomBase* atom)
Construct an OE2DAtomDisplay object that
contains the style information for atom
.
OE2DAtomDisplay(const OE2DAtomDisplay &rhs)
Copy constructor.
CreateCopy¶
OESystem::OEBase *CreateCopy() const
Deep copy constructor that returns a pointer to a copy of the object. The memory returned is dynamically allocated and owned by the caller.
GetCharge¶
int GetCharge() const
Returns the charge rendered next to the atomic label. See example in Figure: Example of charge representation. The number will be rendered in the following ways:
- 0
nothing is rendered
- -1
negative sign “-” is rendered
- 1
positive sign “+” is rendered
- less than -1
“-<n>” is rendered
- greater than 1
“+<n>” is rendered
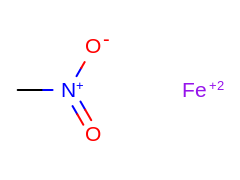
Example of charge representation¶
See also
OE2DAtomDisplay.SetCharge
method
GetCoords¶
const OE2DPoint &GetCoords() const
Returns the coordinates of the atom in the coordinate system that is defined when a OE2DMolDisplay object is constructed with a specific width and height. This is the exact location of the atom when it is rendered into an image.
See also
OE2DAtomDisplay.SetCoords
methodOE2DPoint method
GetHCount¶
unsigned int GetHCount() const
Returns the hydrogen count to render for this atom. See example in Figure: Example of hydrogen count representation. The number will be rendered in the following ways:
- 0
no “H” label is rendered
- 1
“H” is rendered next to the atomic label
- greater than 1
this number is rendered as a sub-script of the “H” label
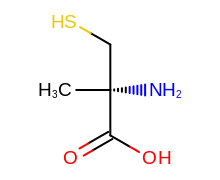
Example of hydrogen count representation¶
See also
OE2DAtomDisplay.SetHCount
methodOE2DAtomDisplay.SetHPosition
method
GetHPosition¶
unsigned int GetHPosition() const
Returns the position of the hydrogen label relative to the
atomic label. The return value is taken from the
OEHydrogenPos
namespace.
See also
OE2DAtomDisplay.SetHPosition
methodOE2DAtomDisplay.SetHCount
methodOEHydrogenPos
namespace
GetLabel¶
const std::string &GetLabel() const
Returns the string that is rendered as the atomic label at the
coordinates returned by the
OE2DAtomDisplay.GetCoords
method.
The color of the font is set by the
See also
OE2DAtomDisplay.SetLabel
method
GetLabelFont¶
const OEFont &GetLabelFont() const
Returns the font that is used when rendering the atomic label string of the OE2DAtomDisplay object.
See also
OE2DAtomDisplay.SetLabelFont
methodOE2DAtomDisplay.SetLabel
methodOEFont class
GetMass¶
unsigned int GetMass() const
Returns the isotope information rendered as a super-script next to the atomic label. If zero, nothing is rendered. See example in Figure: Example of isotope representation.
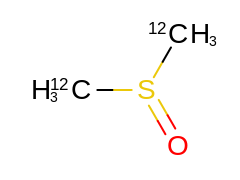
Example of isotope representation¶
See also
OE2DAtomDisplay.SetMass
method
GetProperty¶
const std::string &GetProperty() const
Returns the string rendered next to the atom. If the string is empty, nothing is rendered.
See also
OE2DAtomDisplay.SetProperty
method
GetPropertyFont¶
const OEFont &GetPropertyFont() const
Returns the font that is used when rendering the property string of the OE2DAtomDisplay object.
See also
OE2DAtomDisplay.SetProperty
methodOEFont class
GetPropertyOffset¶
const OE2DPoint &GetPropertyOffset() const
Returns the offset vector relative to the coordinates returned
by OE2DAtomDisplay.GetCoords
that is used
to position the property label of the atom. These vectors are
calculated during OE2DMolDisplay construction
to minimize potentially clashing with the rest of the
depiction.
See Figure: The property offset vector is relative to coordinates returned by
the GetCoords method.
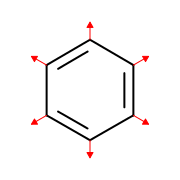
The property offset vector is relative to coordinates returned by the OE2DAtomDisplay::GetCoords method¶
This allows for any string representable information to be rendered at a logical location near the atom. See example in Example of indices as an atom property.
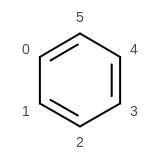
Example of indices as an atom property¶
See also
OE2DPoint class
GetRadical¶
unsigned int GetRadical() const
Returns the radical display type of the atom. The return value
is taken from the OERadicalDisplayType
namespace.
See example in Example of rendering radicals.
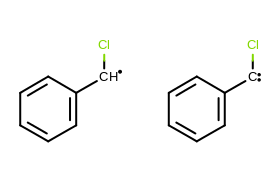
Example of rendering radicals¶
See also
OE2DAtomDisplay.SetRadical
methodOERadicalDisplayType
namespace
HasLabel¶
bool HasLabel() const
Returns whether or not the atomic label string of the OE2DAtomDisplay object is empty.
See also
OE2DAtomDisplay.GetLabel
methodOE2DAtomDisplay.SetLabel
method
HasProperty¶
bool HasProperty() const
Returns whether or not the property string of the OE2DAtomDisplay object is empty.
See also
OE2DAtomDisplay.GetProperty
methodOE2DAtomDisplay.SetProperty
method
SetCharge¶
void SetCharge(int charge)
Sets the charge rendered next to the atomic label.
See also
OE2DAtomDisplay.GetCharge
method
SetCoords¶
void SetCoords(const OE2DPoint &coords)
Sets the exact coordinates of the atom in the rendered image.
See also
OE2DAtomDisplay.GetCoords
methodOE2DPoint method
SetHCount¶
void SetHCount(unsigned int hcount)
Set the hydrogen count to render for this atom.
See also
OE2DAtomDisplay.GetHCount
method for more information on the meaning of various input integers.
SetHPosition¶
void SetHPosition(unsigned int hpos)
Sets the position of hydrogen label relative to the atomic label.
- hpos
This value has to be from the
OEHydrogenPos
namespace.
See also
OE2DAtomDisplay.GetHPosition
methodOEHydrogenPos
namespace
SetLabel¶
void SetLabel(const std::string &label)
Sets the string that is rendered as the “atomic” label at the position
returned by the OE2DAtomDisplay.GetCoords
method.
See also
OE2DAtomDisplay.GetLabel
method
SetLabelFont¶
void SetLabelFont(const OEFont &font)
Sets the font that is used when rendering the atomic label of the OE2DAtomDisplay object.
See also
OE2DAtomDisplay.GetLabelFont
method
SetMass¶
void SetMass(unsigned int mass)
Sets the isotope information rendered next to the atomic label.
See also
OE2DAtomDisplay.GetMass
method
SetProperty¶
void SetProperty(const std::string &label)
Sets the string rendered next to the atom.
Warning
The preferred way to set the atom property strings is to use the
OE2DMolDisplayOptions.SetAtomPropertyFunctor
method.
See example in the Displaying Atom Properties
section.
See also
SetPropertyFont¶
void SetPropertyFont(const OEFont &font)
Sets the font that is used when rendering the property string of the OE2DAtomDisplay object.
Warning
The preferred way to set the font of the atom property strings
is to use the OE2DMolDisplayOptions.SetAtomPropLabelFont
method.
See also
OE2DAtomDisplay.GetProperty
methodOEFont class
SetPropertyOffset¶
void SetPropertyOffset(const OE2DPoint &offset)
Sets the offset vector that is used to position the property label of the atom.
See also
OE2DPoint class
SetRadical¶
void SetRadical(unsigned int radical)
Sets the radical type of the atom.
- radical
This value has to be from the
OERadicalDisplayType
namespace.
See also
OE2DAtomDisplay.GetRadical
methodOERadicalDisplayType
namespace