OEShortestPath¶
Link |
Description |
---|---|
find shortest path between two atoms |
|
find shortest path between two atoms (excluding certain atoms) |
|
find shortest path between two atoms (excluding certain bonds) |
OESystem::OEIterBase<OEAtomBase> *
OEShortestPath(const OEAtomBase *a, const OEAtomBase *b)
Returns a shortest path between the two given atoms. If no path exists
the OEShortestPath
function returns an empty iterator.
Example
mol = oechem.OEGraphMol()
oechem.OESmilesToMol(mol, "c1ccc2c(c1)cccn2")
atomA = mol.GetAtom(oechem.OEHasAtomIdx(2))
atomB = mol.GetAtom(oechem.OEHasAtomIdx(8))
print("shortest path =", end=" ")
for atom in oechem.OEShortestPath(atomA, atomB):
print(str(atom), end=" ")
print("\n")
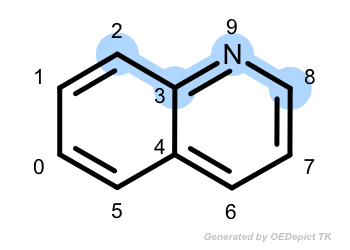
Example of shortest path between atom 2 and 8¶
Note
The shortest path returned by the OEShortestPath
function is
non-unique. There are more than one valid shortest path can exist between two
atoms. The OEShortestPath
only returns one of them.
For example, there are three valid six atoms long shortest path exist between atom
1 and 7 in the example above: 1, 2, 3, 9, 8, 7
, 1, 0, 5, 4, 6, 7
, and
1, 2, 3, 4, 6, 7
.
OESystem::OEIterBase<OEAtomBase> *
OEShortestPath(const OEAtomBase *a, const OEAtomBase *b,
const OESystem::OEUnaryPredicate<OEAtomBase> &excludeatoms)
Returns a shortest path between the two given atoms. The returned path will exclude
any atom that satisfies the given atom predicate.
If no path exists the OEShortestPath
function returns
an empty iterator.
Example
mol = oechem.OEGraphMol()
oechem.OESmilesToMol(mol, "c1ccc2c(c1)cccn2")
atomA = mol.GetAtom(oechem.OEHasAtomIdx(2))
atomB = mol.GetAtom(oechem.OEHasAtomIdx(8))
print("shortest path =", end=" ")
for atom in oechem.OEShortestPath(atomA, atomB, oechem.OEIsNitrogen()):
print(str(atom), end=" ")
print("\n")
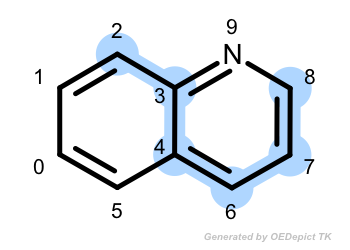
Example of shortest path between atom 2 and 8 without traversing any nitrogen atom¶
OESystem::OEIterBase<OEAtomBase> *
OEShortestPath(const OEAtomBase *a, const OEAtomBase *b,
const OESystem::OEUnaryPredicate<OEBondBase> &excludebonds)
Returns a shortest path between the two given atoms. The returned path will exclude
any bond that satisfies the given bond predicate.
If no path exists, the OEShortestPath
function returns
an empty iterator.