OEHighlightLabel¶
class OEHighlightLabel
The OEHighlightLabel class allows the
customization the style of the label that can be position
on molecule depiction by calling the OEAddLabel
function.
The OEHighlightLabel class stores the following properties:
Property |
Get method |
Set method |
Corresponding namespace / class / type |
---|---|---|---|
text |
string |
||
bounding box pen |
|||
label font |
|||
label font scale |
positive floating point number in the range of |
Constructors¶
OEHighlightLabel(const std::string &text)
Creates an OEHighlightLabel object with default style.
- text
The text that is going to be positioned on the molecule depiction.
Example:
label = oedepict.OEHighlightLabel('match')
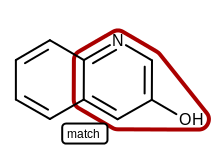
Example of adding label with default style¶
OEHighlightLabel(const std::string &text, const OEFont &font)
Creates an OEHighlightLabel object with user-defined font.
- text
The text that is going to be positioned on the molecule depiction.
- font
The OEFont object that encapsulates properties that determine the display style of text.
See also
OEFont class
Example:
font = oedepict.OEFont(oedepict.OEFontFamily_Courier, oedepict.OEFontStyle_Bold, 12,
oedepict.OEAlignment_Center, oechem.OEDarkRed)
label = oedepict.OEHighlightLabel('match', font)
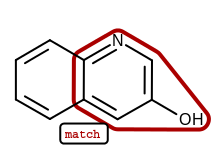
Example of adding label with user-defined font¶
OEHighlightLabel(const std::string &text, const OESystem::OEColor &color)
Creates an OEHighlightLabel object with user-defined border color.
- text
The text that is going to be positioned on the molecule depiction.
- color
The color of the border around the label.
Example:
label = oedepict.OEHighlightLabel('match', oechem.OEDarkRed)
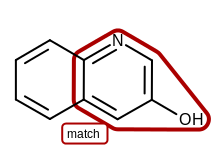
Example of adding label with user-defined border color¶
See also
OEColor class
GetBoundingBoxPen¶
const OEPen &GetBoundingBoxPen() const
Returns the pen that is used to draw a border around the label.
See also
OEPen class
SetBoundingBoxPen
method
GetFont¶
const OEFont &GetFont() const
Returns the font of the label.
See also
OEFont class
OEHighlightLabel.SetFont
method
GetFontScale¶
double GetFontScale() const
Returns the multiplier that can be used to increase or decrease the font size
of the label relative to the font size used to render labels of the
of the depicted molecule.
The default value is 0.75
.
See also
OEFont class
SetBoundingBoxPen¶
void SetBoundingBoxPen(const OEPen &pen)
Sets the pen that is used to draw a border around the label.
Example:
label = oedepict.OEHighlightLabel('match')
pen = oedepict.OEPen(oechem.OEColor(255, 220, 220), oechem.OEDarkRed, oedepict.OEFill_On, 16.0,
oedepict.OEStipple_ShortDash)
label.SetBoundingBoxPen(pen)
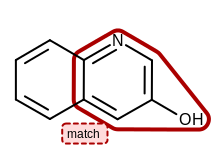
Example of adding label with user-defined border¶
See also
OEPen class
SetFont¶
void SetFont(const OEFont &font)
Set the font of the label.
Example:
label = oedepict.OEHighlightLabel('match')
font = oedepict.OEFont(oedepict.OEFontFamily_Default, oedepict.OEFontStyle_Default, 12,
oedepict.OEAlignment_Center, oechem.OEDarkRed)
label.SetFont(font)
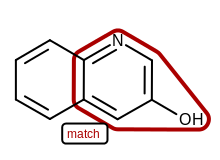
Example of adding label with user-defined font¶
Note
The size of fonts of the label depends on:
the size of the font used to render atom labels of the the depicted molecule
the multiplier set by the
OEHighlightLabel.SetFontScale
method
See also
OEFont class
SetFontScale¶
void SetFontScale(double scale)
Sets the multiplier that can be used to increase or decrease the font size of the label relative to the font size used to render atom labels of the of the depicted molecule.
- scale
This value has to be either
0.0
or in a range of[0.25, 1.50]
. The default value is0.75
.In case of
0.0
, the label is not scaled with the molecule but rather fixed font size is used regardless of the size of the depicted molecule.
Example:
label = oedepict.OEHighlightLabel('match')
label.SetFontScale(1.2)
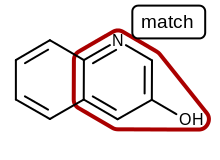
Example of adding label with user-defined font scaling¶