OEAddLabel¶
Adds label to an image or molecule display.
Link |
Description |
---|---|
adding label to an image |
|
adding label to atoms of a match |
|
adding label to set of atoms |
|
adding label to atoms specified by a predicate |
Note
When adding label associated with atoms it is recommended to use the
OEAddLabel
along with the OEAddHighlighting
function for visual clarity.
void OEAddLabel(OEImageBase& image, const OE2DPoint& center,
const OEHighlightLabel& label)
- image
The image in which the label is drawn.
- center
The center of the label on the image.
- label
The OEHighlightLabel object that stores properties that determine the styles of the label along with the text of the label itself.
Example:
private static void OEAddLabel_OEImage(OEImageBase image)
{
OEHighlightLabel label = new OEHighlightLabel("Hello!");
OEDepict.OEAddLabel(image, new OE2DPoint(50, 50), label);
label.SetBoundingBoxPen(OEDepict.OETransparentPen);
OEDepict.OEAddLabel(image, new OE2DPoint(100, 50), label);
label.SetBoundingBoxPen(OEDepict.OELightGreyPen);
OEDepict.OEAddLabel(image, new OE2DPoint(150, 50), label);
}
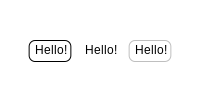
Example of adding labels to an image¶
void OEAddLabel(OE2DMolDisplay &disp,
const OEHighlightLabel &label,
const OEChem::OEMatchBase &match)
Adds label to atoms of an OEMatchBase object that can be initialized by substructure search or maximum common substructure search.
- disp
The OE2DMolDisplay object on which the label is going to be positioned.
- label
The OEHighlightLabel object that stores properties that determine the styles of the label along with the text of the label itself.
- match
The label is positioned based on the target atoms of the OEMatchBase object.
Example:
private static void OEAddLabel_OEMatch(OE2DMolDisplay disp)
{
OESubSearch subs = new OESubSearch("a1aaaaa1");
bool unique = true;
OEHighlightByBallAndStick highlight = new OEHighlightByBallAndStick(OEChem.OELightGreen);
foreach (OEMatchBase match in subs.Match(disp.GetMolecule(), unique))
{
OEDepict.OEAddHighlighting(disp, highlight, match);
OEHighlightLabel label = new OEHighlightLabel("aromatic", OEChem.OELightGreen);
OEDepict.OEAddLabel(disp, label, match);
}
}
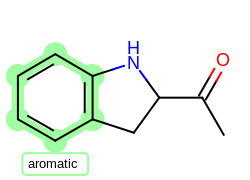
Example of adding label to 6-membered aromatic ring¶
void OEAddLabel(OE2DMolDisplay &disp,
const OEHighlightLabel &label,
const OEChem::OEAtomBondSet &abset)
Adds label to atoms stored in the OEAtomBondSet object.
- disp
The OE2DMolDisplay object on which the label is going to be positioned.
- label
The OEHighlightLabel object that stores properties that determine the styles of the label along with the text of the label itself.
- abset
The label is positioned based on the atoms stored in the OEAtomBondSet object.
Example:
private static void OEAddLabel_OEAtomBondSet(OE2DMolDisplay disp)
{
OEMolBase mol = disp.GetMolecule();
OEAtomBondSet ringset = new OEAtomBondSet(mol.GetAtoms(new OEAtomIsInRing()),
mol.GetBonds(new OEBondIsInRing()));
OEHighlightByBallAndStick ringhighlight = new OEHighlightByBallAndStick(OEChem.OELightGreen);
OEDepict.OEAddHighlighting(disp, ringhighlight, ringset);
OEHighlightLabel ringlabel = new OEHighlightLabel("ring", OEChem.OELightGreen);
OEDepict.OEAddLabel(disp, ringlabel, ringset);
OEAtomBondSet chainset = new OEAtomBondSet(mol.GetAtoms(new OEAtomIsInChain()),
mol.GetBonds(new OEBondIsInChain()));
OEHighlightByBallAndStick chainhighlight = new OEHighlightByBallAndStick(OEChem.OEBlueTint);
OEDepict.OEAddHighlighting(disp, chainhighlight, chainset);
OEHighlightLabel chainlabel = new OEHighlightLabel("chain", OEChem.OEBlueTint);
OEDepict.OEAddLabel(disp, chainlabel, chainset);
}
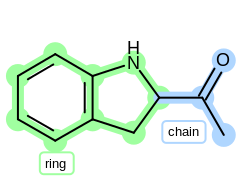
Example of adding label to ring and chain components of a molecule¶
void OEAddLabel(OE2DMolDisplay &disp,
const OEHighlightLabel &label,
const OESystem::OEUnaryPredicate<OEChem::OEAtomBase> &atompred)
Adds label to atoms for which the given atom predicate returns true.
- disp
The OE2DMolDisplay object on which the label is going to be positioned.
- label
The OEHighlightLabel object that stores properties that determine the styles of the label along with the text of the label itself.
- atompred
The label is positioned based on the atoms for which the given atom predicate returns true.
Example:
private static void OEAddLabel_Predicate(OE2DMolDisplay disp)
{
OEHighlightByBallAndStick ringhighlight = new OEHighlightByBallAndStick(OEChem.OELightGreen);
OEDepict.OEAddHighlighting(disp, ringhighlight, new OEAtomIsInRing(), new OEBondIsInRing());
OEHighlightLabel ringlabel = new OEHighlightLabel("ring", OEChem.OELightGreen);
OEDepict.OEAddLabel(disp, ringlabel, new OEAtomIsInRing());
OEHighlightByBallAndStick chainhighlight = new OEHighlightByBallAndStick(OEChem.OEBlueTint);
OEDepict.OEAddHighlighting(disp, chainhighlight, new OEAtomIsInChain(), new OEBondIsInChain());
OEHighlightLabel chainlabel = new OEHighlightLabel("chain", OEChem.OEBlueTint);
OEDepict.OEAddLabel(disp, chainlabel, new OEAtomIsInChain());
}
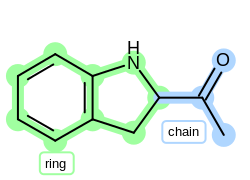
Example of adding label to ring and chain components of a molecule¶