OEImage¶
class OEImage : public OEImageBase
OEImage class is implemented as a display list i.e.
a container of drawing commands.
For example, when the OEImage.DrawLine
method is
called, rather than immediately drawing a line specified by the parameters,
a line drawing operation is created that copies all parameters for later
execution.
When the OEImage.Render
method is invoked, the
drawing commands stored in the OEImage object are
executed in the same order in which they were issued.
The following methods are publicly inherited from OEImageBase:
Constructors¶
OEImage(double width, double height, const OESystem::OEColor& bgColor = OESystem::OEWhite);
Default constructor that creates an OEImage with the specified width and height.
- width, height
The dimensions of the image, both have to be positive (non-zero) numbers.
- bgColor
The color that is used to clear the image upon construction. The default background color is
OEWhite
. A.png
image with transparent background can be generated by passing theOETransparentColor
as the background color.
OEImage(const OEImage &rhs)
Copy constructor.
OEImage(const OEImage &src, double scale)
Copy constructor with scaling.
- scale
The scaling factor is used to create a new image from the given one. The scaling factor has to be a positive (non-zero) number.
The following code snippet shows how to half and double the size of an image.
OEImage image = new OEImage(100.0, 100.0);
image.DrawCircle(OEDepict.OEGetCenter(image), 40.0, OEDepict.OEBlackPen);
image.DrawText(OEDepict.OEGetCenter(image), "circle", OEDepict.OEDefaultFont);
OEDepict.OEDrawBorder(image, OEDepict.OELightGreyPen);
OEDepict.OEWriteImage("image.png", image);
OEImage halfimage = new OEImage(image, 0.5);
OEDepict.OEWriteImage("halfimage.png", halfimage);
OEImage doubleimage = new OEImage(image, 2.0);
OEDepict.OEWriteImage("doubleimage.png", doubleimage);
original image |
scale = 0.5 |
scale = 2.0 |
---|---|---|
![]() |
![]() |
![]() |
Clear¶
void Clear(const OESystem::OEColor &color)
Appends a clear command to the display list of the OEImage object.
See also
OEImageBase.Clear
methodOEColor class
DrawArc¶
void DrawArc(const OE2DPoint ¢er, double bgnAngle, double endAngle,
double radius, const OEPen &pen)
Appends an arc drawing command to the display list of the OEImage object. See example in Figure: Example of drawing an arc.
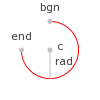
Example of drawing an arc¶
See also
OEImageBase.DrawArc
methodOE2DPoint class
OEPen class
DrawCircle¶
void DrawCircle(const OE2DPoint ¢er, double radius, const OEPen &pen)
Appends a circle drawing command to the display list of the OEImage object. See example in Figure: Example of drawing a circle.
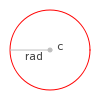
Example of drawing a circle¶
See also
OEImageBase.DrawCircle
methodOE2DPoint class
OEPen class
DrawCubicBezier¶
void DrawCubicBezier(const OE2DPoint &bgn, const OE2DPoint &c1,
const OE2DPoint &c2, const OE2DPoint &end, const OEPen &pen)
Appends a cubic Bézier curve drawing command to the display list of the OEImage object. See example in Figure: Example of drawing a cubic Bezier curve.
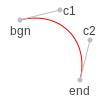
Example of drawing a cubic Bezier curve¶
See also
OEImageBase.DrawCubicBezier
methodOE2DPoint class
OEPen classes
DrawLine¶
void DrawLine(const OE2DPoint &bgn, const OE2DPoint &end, const OEPen &pen)
Appends a line drawing command to the display list of the OEImage object. See example in Figure: Example of drawing a line.
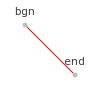
Example of drawing a line¶
See also
OEImageBase.DrawLine
methodOE2DPoint class
OEPen class
DrawPath¶
void DrawPath(const OE2DPath& path, const OEPen& pen);
Appends a path drawing command to the display list of the OEImage object. See example in Figure: Example of drawing a path.
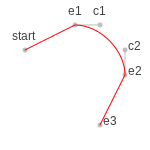
Example of drawing a path¶
See also
OEImageBase.DrawPath
methodOE2DPath class
OEPen class
DrawPie¶
void DrawPie(const OE2DPoint ¢er, double bgnAngle, double endAngle,
double radius, const OEPen &pen)
Appends a pie drawing command to the display list of the OEImage object. See example in Figure: Example of drawing a pie.
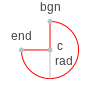
Example of drawing a pie¶
See also
OEImageBase.DrawPie
methodOE2DPoint class
OEPen class
DrawPoint¶
void DrawPoint(const OE2DPoint &p, const OESystem::OEColor &color)
Appends a point drawing command to the display list of the OEImage object. See example in Figure: Example of drawing a point.
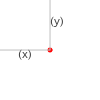
Example of drawing a point¶
See also
OEImageBase.DrawPoint
methodOE2DPoint class
OEColor class
DrawPolygon¶
void DrawPolygon(const std::vector<OE2DPoint> &points, const OEPen &pen)
Appends a polygon drawing command to the display list of the OEImage object. See example in Figure: Example of drawing a polygon.
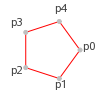
Example of drawing a polygon¶
See also
OEImageBase.DrawPolygon
methodOE2DPoint class
OEPen class
DrawQuadraticBezier¶
void DrawQuadraticBezier(const OE2DPoint &bgn, const OE2DPoint &c,
const OE2DPoint &end, const OEPen &pen)
Appends a quadratic Bézier curve drawing command to the display list of the OEImage object. See example in Figure: Example of drawing a quadratic Bezier curve.
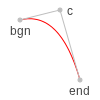
Example of drawing a quadratic Bezier curve¶
DrawRectangle¶
void DrawRectangle(const OE2DPoint &tl, const OE2DPoint &br, const OEPen &pen)
Appends a polygon drawing command to the display list of the OEImage object. See example in Figure: Example of drawing a rectangle.
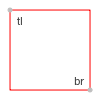
Example of drawing a rectangle¶
See also
OEImageBase.DrawRectangle
methodOE2DPoint class
OEPen class
DrawText¶
void DrawText(const OE2DPoint &c, const std::string &text, const OEFont &font,
double maxwidth=0.0)
Appends a text drawing command to the display list of the OEImage object. See example in Figure: Example of drawing a text.
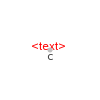
Example of drawing a text¶
See also
OEImageBase.DrawText
methodOE2DPoint class
OEFont class
DrawTriangle¶
void DrawTriangle(const OE2DPoint &a, const OE2DPoint &b, const OE2DPoint &c,
const OEPen &pen)
Appends a triangle drawing command to the display list of the OEImage object. See example in Figure: Example of drawing a triangle.
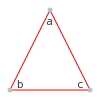
Example of drawing a triangle¶
See also
OEImageBase.DrawTriangle
methodOE2DPoint class
OEPen class