OEReport¶
class OEReport
This class represents a layout manager that allows generation of multi-page images with two types of page layouts:
- body
The body page layout provides a convenient way to position information in the middle of the page relative to the page footers and headers. For example, see the \(1^{st}\) page in Figure: Schematic representation of documents that can be created using the OEReport class.
- grid
The grid page layout provides a convenient way to organize information (such as text and molecule depiction) into rows and columns. For example, see the \(2^{nd}\) and \(3^{rd}\) pages in Figure: Schematic representation of documents that can be created using the OEReport class.
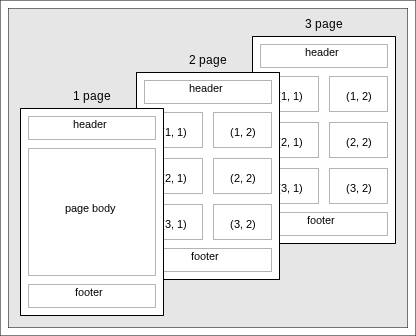
Schematic representation of documents that can be created using the OEReport class¶
See also
Multi Page Reports section
OEReportOptions class
OEWriteReport
functionOEWriteReportToString
function
Constructors¶
OEReport(const OEReportOptions &opts)
Initializes an OEReport object using the given options.
- opts
The OEReportOptions object that stores parameters determining the layout of the body and the grid pages of the report.
OEReport(unsigned int rowsperpage, unsigned int colsperpage)
Initializes an OEReport object with the given parameters.
- rowsperpage
The number of rows on each grid layout page.
- colsperpage
The number of columns on each grid layout page.
Note
When a report is initialized with only the number of rows and columns per page parameter, all other parameters defining the layout of the pages will be initialized from the default constructor of the OEReportOptions class.
See also
OEReportOptions class
GetBodies¶
OESystem::OEIterBase<OEImageBase> *GetBodies()
Returns an iterator over all page bodies that have been created by
calling the OEReport.NewBody
method.
Note
If no page has been created by the OEReport.NewBody
method, then the OEReport.GetBodies
method returns
an empty iterator.
See example in Figure: Example of accessing all page bodies of the report, where body areas in the \(1^{st}\) and \(5^{th}\) page are returned.
foreach (OEImageBase body in report.GetBodies())
page 1 |
page 2 |
page 3 |
page 4 |
page 5 |
---|---|---|---|---|
![]() |
![]() |
![]() |
![]() |
![]() |
See also
OEReport.GetBody
methodOEReport.NewBody
method
GetBody¶
OEImageBase *GetBody(unsigned int p)
Returns the OEImageBase pointer of the body area of the \(p^{th}\) page.
- p
This value has to be in the range from 1 to
OEReport.NumPages()
.
Note
If the \(p^{th}\) page of the report was not created by
the OEReport.NewBody
method or the \(p\)
page index is out of range, then the OEReport.GetBody
method returns a NULL pointer.
See also
OEReport.GetBodies
methodOEReport.NewBody
method
GetCell¶
OEImageBase *GetCell(unsigned int p, unsigned int r, unsigned int c)
Returns the OEImageBase pointer for the cell positioned in the \(r^{th}\) row and \(c^{th}\) columns on the \(p^{th}\) page.
- p
This value has to be in the range from 1 to
OEReport.NumPages()
.- r
This value has to be in the range from 1 to
OEReport.NumRowsPerPage()
.- c
This value has to be in the range from 1 to
OEReport.NumColsPerPage()
.
Note
If the cell positioned in the \(r^{th}\) row and
\(c^{th}\) columns on the \(p^{th}\) page has not been
created by the OEReport.NewCell
method,
then the OEReport.GetCell
method returns
a NULL pointer.
See also
OEReport.GetCells
methodOEReport.NewCell
method
GetCellHeight¶
double GetCellHeight() const
Returns the height of the cells on the grid page of the OEReport object.
GetCellWidth¶
double GetCellWidth() const
Returns the width of the cells on the grid page of the OEReport object.
GetCells¶
OESystem::OEIterBase<OEImageBase> *GetCells(unsigned int page=0,
unsigned int row=0,
unsigned int col=0)
Returns an iterator over cells that have been created by calling the
OEReport.NewCell
method.
The cells are returned in the order in which they have been created
i.e. from left to right and top to bottom order on each grid page.
- page
This value has to be in the range from 0 to
OEReport.NumPages()
.- row
This value has to be in the range from 0 to
OEReport.NumRowsPerPage()
.- col
This value has to be in the range from 0 to
OEReport.NumColsPerPage()
.
Example (Figure: Example of accessing all cells of the report)
foreach (OEImageBase cell in report.GetCells())
page 1 |
page 2 |
page 3 |
page 4 |
page 5 |
---|---|---|---|---|
![]() |
![]() |
![]() |
![]() |
![]() |
Example (Figure: Example of accessing all cells on the given row on each page)
uint row = 2;
foreach (OEImageBase cell in report.GetCells(0, row, 0))
page 1 |
page 2 |
page 3 |
page 4 |
page 5 |
---|---|---|---|---|
![]() |
![]() |
![]() |
![]() |
![]() |
Example (Figure: Example of accessing all cells on the given column on each page)
uint col = 2;
foreach (OEImageBase cell in report.GetCells(0, 0, col))
page 1 |
page 2 |
page 3 |
page 4 |
page 5 |
---|---|---|---|---|
![]() |
![]() |
![]() |
![]() |
![]() |
Example (Figure: Example of accessing all cells on the given row and column on each page)
uint row = 2;
uint col = 2;
foreach (OEImageBase cell in report.GetCells(0, row, col))
page 1 |
page 2 |
page 3 |
page 4 |
page 5 |
---|---|---|---|---|
![]() |
![]() |
![]() |
![]() |
![]() |
Example (Figure: Example of accessing all cells on the given row and page)
uint page = 3;
uint row = 2;
foreach (OEImageBase cell in report.GetCells(page, row, 0))
page 1 |
page 2 |
page 3 |
page 4 |
page 5 |
---|---|---|---|---|
![]() |
![]() |
![]() |
![]() |
![]() |
Example (Figure: Example of accessing all cells on the given column and page)
uint page = 3;
uint col = 2;
foreach (OEImageBase cell in report.GetCells(page, 0, col))
page 1 |
page 2 |
page 3 |
page 4 |
page 5 |
---|---|---|---|---|
![]() |
![]() |
![]() |
![]() |
![]() |
Example (Figure: Example of accessing a cell on the given row, column and page)
uint page = 3;
uint row = 2;
uint col = 2;
foreach (OEImageBase cell in report.GetCells(page, row, col))
page 1 |
page 2 |
page 3 |
page 4 |
page 5 |
---|---|---|---|---|
![]() |
![]() |
![]() |
![]() |
![]() |
See also
OEReport.GetCell
methodOEReport.NewCell
method
GetHeader¶
OEImageBase *GetHeader(unsigned int p)
Returns the OEImageBase pointer of the header on the \(p^{th}\) page.
- p
This value has to be in the range from 1 to
OEReport.NumPages()
.
Note
If the OEReport object was initialized with header
height 0.0 or the \(p\) page index is out of range, then the
OEReport.GetHeader
method returns a NULL pointer.
See also
OEReport.GetHeaderHeight
methodOEReport.GetHeaderWidth
methodOEReport.GetHeaders
method
GetHeaderHeight¶
double GetHeaderHeight() const
Returns the height of the header at the top of each page.
See also
OEReport.GetHeaderWidth
method
GetHeaderWidth¶
double GetHeaderWidth() const
Returns the width of the header at the top of each page.
See also
OEReportOptions.GetHeaderHeight
methods
GetHeaders¶
OESystem::OEIterBase<OEImageBase> *GetHeaders()
Returns an iterator over all headers of the OEReport object.
Note
If the OEReport object was initialized with header
height 0.0, then the OEReport.GetHeaders
method
returns an empty iterator.
Example (Figure: Example of accessing all page headers of the report)
foreach (OEImageBase header in report.GetHeaders())
page 1 |
page 2 |
page 3 |
page 4 |
page 5 |
---|---|---|---|---|
![]() |
![]() |
![]() |
![]() |
![]() |
See also
OEReport.GetHeader
methodOEReport.GetHeaderHeight
methodOEReport.GetHeaderWidth
method
GetOptions¶
const OEReportOptions &GetOptions() const
Returns the options used to initialize the OEReport object.
See also
OEReportOptions class
GetPage¶
OEImageBase *GetPage(unsigned int p)
Returns the OEImageBase pointer of the \(p^{th}\) page.
- p
This value has to be in the range from 1 to
OEReport.NumPages()
.
Note
If \(p\) page index is out of range, then the
OEReport.GetPage
method returns a NULL
pointer.
See also
OEReport.GetPages
method
GetPageWidth¶
double GetPageWidth() const
Returns the width of the pages of the report.
See also
OEReportOptions.SetPageWidth
method
GetPages¶
OESystem::OEIterBase<OEImageBase> *GetPages()
Returns an iterator over all the pages of the OEReport object.
See also
OEReport.GetPage
method
IsBodyPage¶
bool IsBodyPage(unsigned int p) const
Returns whether the \(p^{th}\) page of the report
is created by calling the OEReport.NewBody
method.
- p
This value has to be in the range from 1 to
OEReport.NumPages()
.
For example, the OEReport.IsBodyPage
method
returns true for the \(1^{st}\) and \(5^{th}\) pages of the report
shown in Figure: Example of report layout.
page 1 |
page 2 |
page 3 |
page 4 |
page 5 |
|
---|---|---|---|---|---|
![]() |
![]() |
![]() |
![]() |
![]() |
|
IsBodyPage |
true |
false |
false |
false |
true |
See also
OEReport.IsGridPage
methodOEReport.NewBody
method
IsGridPage¶
bool IsGridPage(unsigned int p) const
Returns whether the \(p^{th}\) page of the report
is created by calling the OEReport.NewCell
method.
- p
This value has to be in the range from 1 to
OEReport.NumPages()
.
For example, the OEReport.IsGridPage
method returns
true for the \(2^{nd}\), \(3^{rd}\), and \(4^{th}\) pages
of the report shown in
Figure: Example of report layout.
page 1 |
page 2 |
page 3 |
page 4 |
page 5 |
|
---|---|---|---|---|---|
![]() |
![]() |
![]() |
![]() |
![]() |
|
IsGridPage |
false |
true |
true |
true |
false |
See also
OEReport.IsBodyPage
methodOEReport.NewCell
method
NewBody¶
OEImageBase *NewBody()
Creates a new body layout page and returns the area between the header and the footer. See example in Figure: Example of creating a new body page.
Note
The height and width of the returned area depend on the options with which the OEReport object was constructed: page margins, gap between the cells, and the height of the header and footer.
page 1 |
page 2 |
page 3 |
---|---|---|
![]() |
![]() |
![]() |
See also
OEReport.IsBodyPage
methodOEReport.GetBody
methodOEReport.GetBodies
method
NewCell¶
OEImageBase *NewCell()
Creates and returns a new cell. If the last page of the report is a grid page, then a new cell is created from left to right and top to bottom order. If no more cells are left on the grid page or the last page is a body page, then a new grid page is created before returning the first cell of this new page. See example in Figure: Example of creating a new cell.
Note
The cells of a grid page are equal-sized and evenly spaced. The height and width of the cells depend on the options with which the OEReport object was constructed: the number of rows and columns per page, page margins, gap between the cells, and the height of the header and footer.
page 1 |
page 2 |
page 3 |
---|---|---|
![]() |
![]() |
![]() |
See also
OEReport.IsGridPage
methodOEReport.GetCell
methodOEReport.GetCells
method
NumBodyPages¶
unsigned int NumBodyPages() const
Returns the number of body pages of the OEReport
object, i.e. the number of pages for which the
OEReport.IsBodyPage
method returns true.
See also
OEReport.IsBodyPage
methodOEReport.NumGridPages
methodOEReport.NumPages
method
NumColsPerPage¶
unsigned int NumColsPerPage() const
Returns the number of columns on each grid page of the OEReport object.
See also
OEReport.NumRowsPerPage
method
NumGridPages¶
unsigned int NumGridPages() const
Returns the number of grid pages of the OEReport
object, i.e. the number of pages for which the
OEReport.IsGridPage
method returns true.
See also
OEReport.IsGridPage
methodOEReport.NumBodyPages
methodOEReport.NumPages
method
NumPages¶
unsigned int NumPages() const
Returns the number of pages of the OEReport
object. This is the sum of the numbers returned by the
OEReport.NumBodyPages
and
OEReport.NumGridPages
methods.
See also
OEReport.NumBodyPages
methodOEReport.NumGridPages
method
NumRowsPerPage¶
unsigned int NumRowsPerPage() const
Returns the number of rows on each grid page of the OEReport object.
See also
OEReport.NumColsPerPage
method