OEReportOptions¶
class OEReportOptions
This class represents the OEReportOptions class that encapsulates properties that determine the layout of a OEReport object.
See Figure: Schematic representation of documents that can be created using the OEReport class.
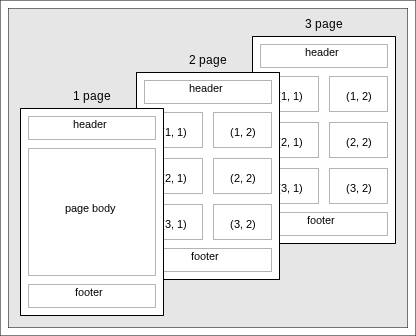
Schematic representation of documents that can be created using the OEReport class¶
The OEReportOptions class stores the following properties:
Property
Get method
Set method
Corresponding namespace / class / type
rows per page
set upon construction
integer greater than 0
columns per page
set upon construction
integer greater than 0
page width
floating point number /
OEPageSize
/OEPageOrientation
page height
floating point number /
OEPageSize
/OEPageOrientation
page margins
cell gap
floating point number
header height
floating point number
header width
N/A
floating point number
footer height
floating point number
footer width
N/A
floating point number
See also
OEReport class
Constructors¶
OEReportOptions(unsigned int rowsperpage = 3, unsigned int colsperpage = 2)
- rowsperpage
The number of rows on each grid page of the report.
- colsperpage
The number of columns on each grid page of the report.
Note
Both the number of rows and columns per page have to be greater than 0.
Property |
Default value |
---|---|
rows per page |
3 |
columns per page |
2 |
page width |
|
page height |
|
margins |
|
cell gap |
5.0 |
header height |
0.0 (no header is generated by default) |
footer height |
0.0 (no footer is generated by default) |
Example (Figure: Example of report generation with default options)
OEReportOptions opts = new OEReportOptions(3, 2);
![]() |
![]() |
OEReportOptions(const OEReportOptions &rhs)
Copy constructor.
GetCellGap¶
double GetCellGap() const
Returns the space between the cells of the report.
See also
OEReportOptions.SetCellGap
method
GetCellHeight¶
double GetCellHeight() const
Returns the height of the cells on the grid pages of the report.
Note
The height of the cells depends on the number of rows per page, the top and bottom margins, header and footer heights, and the gap between the cells
GetCellWidth¶
double GetCellWidth() const
Returns the width of the cells on the grid pages of the report.
Note
The width of the cells depends on the number of columns per page, the left and right margins, and the gap between the cells.
GetHeaderHeight¶
double GetHeaderHeight() const
Returns the height of the header at the top of each page.
See also
GetHeaderWidth¶
double GetHeaderWidth() const
Returns the width of the header at the top of each page.
GetPageMargin¶
double GetPageMargin(unsigned int margin) const
Returns the size of a specific page margin of the report.
- margin
This value has to be from the
OEMargin
namespace.
See also
GetPageWidth¶
double GetPageWidth() const
Returns the width of the page of the report.
See also
OEReportOptions.SetPageWidth
method
NumColsPerPage¶
unsigned int NumColsPerPage() const
Returns the number of columns on each grid page of the report.
See also
NumRowsPerPage¶
unsigned int NumRowsPerPage() const
Returns the number of rows on each grid page of the report.
See also
SetCellGap¶
void SetCellGap(double size)
Sets the space between the cells of the report.
- size
This value has to be a non-negative number.
Example (Figure: Example of using the SetCellGap method)
OEReportOptions opts = new OEReportOptions(3, 2);
opts.SetCellGap(100);
![]() |
![]() |
See also
OEReportOptions.GetCellGap
method
SetHeaderHeight¶
void SetHeaderHeight(double height)
Sets the height of the header at the top of each page.
- height
This value has to be a non-negative number.
Note
0.0 means that a header is not going to be created on the pages.
Example (Figure: Example of using the SetHeaderHeight method)
OEReportOptions opts = new OEReportOptions(3, 2);
opts.SetHeaderHeight(100);
![]() |
![]() |
See also
SetPageHeight¶
void SetPageHeight(double height)
Sets the height of the pages of the report.
- height
This number has to be greater than 0.0.
Example (Figure: Example of using the SetPageHeight method)
OEReportOptions opts = new OEReportOptions(3, 2);
opts.SetPageHeight(opts.GetPageHeight()*0.50);
![]() |
![]() |
See also
OEReportOptions.SetPageSize
methodOEReportOptions.GetPageWidth
method
SetPageMargin¶
void SetPageMargin(unsigned int margin, double size)
Sets the size of a specific page margin of the report.
- margin
This value has to be from the
OEMargin
namespace.- size
This value has to be a non-negative number.
Example (Figure: Example of using the SetPageMargin method)
OEReportOptions opts = new OEReportOptions(3, 2);
opts.SetPageMargin(OEMargin.Left, 100);
opts.SetPageMargin(OEMargin.Right, 100);
![]() |
![]() |
See also
SetPageMargins¶
void SetPageMargins(double size)
Sets the size of all the margins of the report.
- size
This value has to be a non-negative number.
Example (Figure: Example of using the SetPageMargins method)
OEReportOptions opts = new OEReportOptions(3, 2);
opts.SetPageMargins(100);
![]() |
![]() |
See also
SetPageOrientation¶
void SetPageOrientation(unsigned int orientation)
Sets the orientation of the pages of the report.
- orientation
This value has to be from the
OEPageOrientation
namespace.
Example (Figure: Example of using the SetPageOrientation method)
OEReportOptions opts = new OEReportOptions(3, 2);
opts.SetPageOrientation(OEPageOrientation.Landscape);
![]() |
![]() |
See also
OEReportOptions.SetPageSize
methodOEReportOptions.SetPageWidth
method
SetPageSize¶
void SetPageSize(unsigned int pagesize)
Sets the width and height of the pages of the report.
- pagesize
This value has to be from the
OEPageSize
namespace.
Example (Figure: Example of using the SetPageSize method)
OEReportOptions opts = new OEReportOptions(3, 2);
opts.SetPageSize(OEPageSize.US_Legal);
![]() |
![]() |
See also
OEReportOptions.SetPageWidth
method
SetPageWidth¶
void SetPageWidth(double width)
Sets the width of the pages of the report.
- width
This number has to be greater than 0.0.
Example (Figure: Example of using the SetPageWidth method)
OEReportOptions opts = new OEReportOptions(3, 2);
opts.SetPageWidth(opts.GetPageWidth()*0.50);
![]() |
![]() |
See also
OEReportOptions.SetPageSize
method