OEAddHighlighting¶
Highlights atoms and/or bonds of the displayed molecule.
Link |
Description |
---|---|
highlights atoms with OEHighlightBase |
|
highlights bonds with OEHighlightBase |
|
highlights atoms and bonds with OEHighlightBase |
|
highlights atoms and bonds of a match with OEHighlightBase |
|
highlights atoms and bonds of a set with OEHighlightBase |
|
highlights atoms and bonds of substructure matches OEHighlightBase |
Link |
Description |
---|---|
highlights atoms with color and style |
|
highlights bonds with color and style |
|
highlights atoms and bonds with color and style |
|
highlights atoms and bonds of a match with color and style |
|
highlights atoms and bonds of a set with color and style |
|
highlights atoms and bonds of substructure matches |
Parameters :
- disp
The OE2DMolDisplay object of which atoms add/or bonds being highlighted.
- highlight
The OEHighlightBase object that specifies the style of the highlighting.
- color
The OEColor object that specifies the color of the highlighting.
- style
The style of the highlighting from the
OEHighlightStyle
namespace.- atompred
OEAddHighlighting
function highlights only atoms for which the given atom predicate returns true.- bondpred
OEAddHighlighting
function highlights only bonds for which the given bond predicate returns true.- match
OEAddHighlighting
function highlights the target atoms and bonds of the OEMatchBase object.- abset
Stores the atoms and bonds being highlighted.
- subsearch
The substructure search object of the matches being highlighted.
Highlights atom and/or bonds with the style implemented in the given OEHighlightBase object
The atoms and bonds being highlighted can be specified either by a predicate or an OEMatchBase object or an OEAtomBondSet object.
The following code snippets show different ways to highlight any 6 membered aromatic ring in a molecule using the OEHighlightByBallAndStick highlighting class. They all generate the image shown in Figure: Example of highlighting 6-membered aromatic rings.
Highlighting using atom and bond predicates:
void OEAddHighlighting(OE2DMolDisplay &disp, const OEHighlightBase &highlight, const OESystem::OEUnaryPredicate<OEChem::OEAtomBase> &atompred)
Highlights atoms with the style implemented in the given OEHighlightBase object. The atoms being highlighted are specified by given atom predicate.
void OEAddHighlighting(OE2DMolDisplay &disp, const OEHighlightBase &highlight, const OESystem::OEUnaryPredicate<OEChem::OEBondBase> &bondpred)
Highlights bonds with the style implemented in the given OEHighlightBase object. The bonds being highlighted are specified by given bond predicates.
void OEAddHighlighting(OE2DMolDisplay& disp, const OEHighlightBase& highlight, const OESystem::OEUnaryPredicate<OEChem::OEAtomBase> &atompred, const OESystem::OEUnaryPredicate<OEChem::OEBondBase> &bondpred)
Highlights atoms and bonds with the style implemented in the given OEHighlightBase object. The atoms and bonds being highlighted are specified by given atom and bond predicates, respectively.
Example: (See image generated in Example of highlighting 6-membered aromatic rings)
class Pred6MemAromAtom(oechem.OEUnaryAtomPred): def __call__(self, atom): return atom.IsAromatic() and oechem.OEAtomIsInAromaticRingSize(atom, 6) class Pred6MemAromBond(oechem.OEUnaryBondPred): def __call__(self, bond): return bond.IsAromatic() and oechem.OEBondIsInAromaticRingSize(bond, 6) def OEAddHighlighting_Predicate(disp): highlightstyle = oedepict.OEHighlightByBallAndStick(oechem.OELightGreen) oedepict.OEAddHighlighting(disp, highlightstyle, Pred6MemAromAtom()) oedepict.OEAddHighlighting(disp, highlightstyle, Pred6MemAromBond()) def OEAddHighlighting_AtomAndBondPredicate(disp): highlightstyle = oedepict.OEHighlightByBallAndStick(oechem.OELightGreen) oedepict.OEAddHighlighting(disp, highlightstyle, Pred6MemAromAtom(), Pred6MemAromBond())
Highlighting using an OEMatchBase object that is initialized by substructure search or maximum common substructure search:
void OEAddHighlighting(OE2DMolDisplay &disp, const OEHighlightBase &highlight, const OEChem::OEMatchBase &match)
Example: (See image generated in Example of highlighting 6-membered aromatic rings)
def OEAddHighlighting_OEMatch(disp): subs = oechem.OESubSearch("a1aaaaa1") unique = True highlightstyle = oedepict.OEHighlightByBallAndStick(oechem.OELightGreen) for match in subs.Match(disp.GetMolecule(), unique): oedepict.OEAddHighlighting(disp, highlightstyle, match)
Highlighting using an OEAtomBondSet object that stores the atoms and bonds being highlighted:
void OEAddHighlighting(OE2DMolDisplay &disp, const OEHighlightBase &highlight, const OEChem::OEAtomBondSet &abset)
Example: (See image generated in Example of highlighting 6-membered aromatic rings)
def OEAddHighlighting_OEAtomBondSet(disp): mol = disp.GetMolecule() abset = oechem.OEAtomBondSet(mol.GetAtoms(Pred6MemAromAtom()), mol.GetBonds(Pred6MemAromBond())) highlightstyle = oedepict.OEHighlightByBallAndStick(oechem.OELightGreen) oedepict.OEAddHighlighting(disp, highlightstyle, abset)
Highlighting using an OESubSearch of which matches are being highlighted:
void OEAddHighlighting(OE2DMolDisplay &disp, const OEHighlightBase &highlight, const OEChem::OESubSearch &subsearch)
Example: (See image generated in Example of highlighting 6-membered aromatic rings)
def OEAddHighlighting_OESubSearch(disp): subsearch = oechem.OESubSearch("a1aaaaa1") highlightstyle = oedepict.OEHighlightByBallAndStick(oechem.OELightGreen) oedepict.OEAddHighlighting(disp, highlightstyle, subsearch)
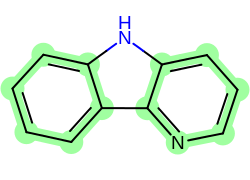
Example of highlighting 6-membered aromatic rings¶
See also
OEHighlightByColor class
OEHighlightByCogwheel class
OEHighlightByStick class
OEHighlightByLasso class
OEDrawHighlighting
functionHighlighting chapter
Highlights atoms and/or bonds with the given color and style
The atoms and bonds being highlighted can be specified either by a predicate or an OEMatchBase object or an OEAtomBondSet object.
The following code snippets show three different ways to highlight any 6 membered aromatic ring in a molecule using the built-in highlighting styles. They all generate the image shown in Figure: Example of highlighting 6-membered aromatic rings.
Highlighting using atom and bond predicates:
void OEAddHighlighting(OE2DMolDisplay &disp, const OESystem::OEColor &color, unsigned int style, const OESystem::OEUnaryPredicate<OEChem::OEAtomBase> &atompred)
Highlights atoms with the given color and style. The atoms being highlighted are specified by given atom predicate.
void OEAddHighlighting(OE2DMolDisplay &disp, const OESystem::OEColor &color, unsigned int style, const OESystem::OEUnaryPredicate<OEChem::OEBondBase> &bondpred)
Highlights bonds with the given color and style. The bonds being highlighted are specified by given bonds predicate.
void OEAddHighlighting(OE2DMolDisplay& disp, const OESystem::OEColor& color, unsigned int style, const OESystem::OEUnaryPredicate<OEChem::OEAtomBase> &atompred, const OESystem::OEUnaryPredicate<OEChem::OEBondBase> &bondpred)
Highlights atoms and bonds with the given color and style. The atoms and bonds being highlighted are specified by given atom and bond predicates, respectively.
Example: (See image generated in Example of highlighting 6-membered aromatic rings)
class Pred6MemAromAtom(oechem.OEUnaryAtomPred): def __call__(self, atom): return atom.IsAromatic() and oechem.OEAtomIsInAromaticRingSize(atom, 6) class Pred6MemAromBond(oechem.OEUnaryBondPred): def __call__(self, bond): return bond.IsAromatic() and oechem.OEBondIsInAromaticRingSize(bond, 6) def OEAddHighlighting_Predicate(disp): oedepict.OEAddHighlighting(disp, oechem.OEDarkGreen, oedepict.OEHighlightStyle_Color, Pred6MemAromAtom()) oedepict.OEAddHighlighting(disp, oechem.OEDarkGreen, oedepict.OEHighlightStyle_Color, Pred6MemAromBond()) def OEAddHighlighting_AtomAndBondPredicate(disp): oedepict.OEAddHighlighting(disp, oechem.OEDarkGreen, oedepict.OEHighlightStyle_Color, Pred6MemAromAtom(), Pred6MemAromBond())
Highlighting using an OEMatchBase object that is initialized by substructure search or maximum common substructure search:
void OEAddHighlighting(OE2DMolDisplay &disp, const OESystem::OEColor &color, unsigned int style, const OEChem::OEMatchBase &match)
Example: (See image generated in Example of highlighting 6-membered aromatic rings)
def OEAddHighlighting_OEMatch(disp): subs = oechem.OESubSearch("a1aaaaa1") unique = True for match in subs.Match(disp.GetMolecule(), unique): oedepict.OEAddHighlighting(disp, oechem.OEDarkGreen, oedepict.OEHighlightStyle_Color, match)
Highlighting using an OEAtomBondSet object that stores the atoms and bonds being highlighted:
void OEAddHighlighting(OE2DMolDisplay &disp, const OESystem::OEColor &color, unsigned int style, const OEChem::OEAtomBondSet &abset)
Example: (See image generated in Example of highlighting 6-membered aromatic rings)
def OEAddHighlighting_OEAtomBondSet(disp): abset = oechem.OEAtomBondSet(mol.GetAtoms(Pred6MemAromAtom()), mol.GetBonds(Pred6MemAromBond())) oedepict.OEAddHighlighting(disp, oechem.OEDarkGreen, oedepict.OEHighlightStyle_Color, abset)
Highlighting using an OESubSearch of which matches are being highlighted:
void OEAddHighlighting(OE2DMolDisplay &disp, const OESystem::OEColor& color, unsigned int style, const OEChem::OESubSearch &subsearch);Example: (See image generated in Example of highlighting 6-membered aromatic rings)
def OEAddHighlighting_OESubSearch(disp): subsearch = oechem.OESubSearch("a1aaaaa1") oedepict.OEAddHighlighting(disp, oechem.OEDarkGreen, oedepict.OEHighlightStyle_Color, subsearch)
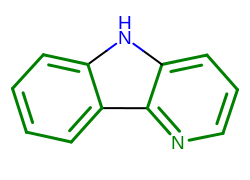
Example of highlighting 6-membered aromatic rings¶
See also
OEColor class
OEHighlightStyle
namespaceHighlighting chapter