Scripting¶
VIDA is a scriptable application which contains a built-in Python interpreter. Python is an interactive, interpreter, strongly and dynamically typed language. More information about Python can be obtained from the Python website. The website contains many useful tutorials and other related information.
All user interaction with the application goes through a Python layer even if the user is not aware of it. For instance, every button in VIDA is associated with a Python command that is sent to the internal interpreter when that button is pressed. The same integration is true of all the menu items and other GUI controls in VIDA.
Journal File¶
The journal file is a recording of every action that is performed within a given session of VIDA. In fact, the journal file is itself a Python script which can be run to regenerate the state of the application at the time the file was written.
The journal file is written to journal.py
in the user directory (see
user_dir). In the unlikely event that VIDA were to quit
unexpectedly, the journal file corresponding to that run will be saved in the
same directory but with a different name containing a time stamp associated
with when the application was started (e.g. journal_WedMar181507132009.py
).
Journal files are particularly useful for debugging unexpected application
behavior and are of extreme benefit to the application developers when
tracking user-reported bugs. The inclusion of journal files (if possible) with
bug reports is greatly encouraged.
Startup File¶
The startup file is a Python script located in the user directory (see
user_dir) named startup.py
. This script (if present) is executed
each time VIDA starts.
The startup file provides the user with a convenient mechanism to customize the appearance and functionality of VIDA using Python.
Scripts Directory¶
In addition to interpreting the startup file (see Startup File)
at runtime, VIDA also executes any Python scripts contained in the
scripts
directory of the user directory (see user_dir). This
capability provides a simple mechanism to quickly and easily add or remove
custom functionality to VIDA without having to change the startup file.
Scripting API¶
The complete details of the VIDA Python interface and API are fully described in the appendix.
Scripting Window¶
The scripting window provides an interactive user interface to the embedded Python interpreter. It also displays the output from any Python commands issued to VIDA as well as any warnings or errors reported by the application.
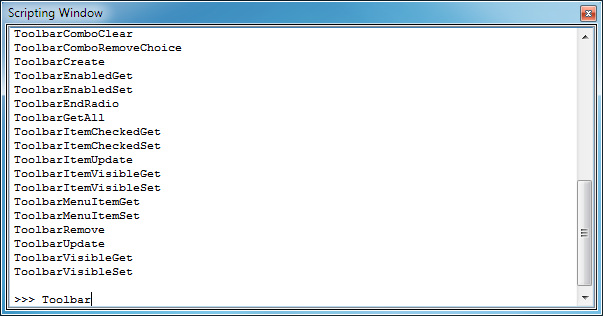
Scripting Window¶
The scripting window supports tab completion of the built-in API methods and hitting the tab key again after tab completion will display the API documentation associated with the command directly in the window.
The window history can be cleared by typing the following command
InterpreterClear()
into the scripting window.
OpenEye Toolkits¶
VIDA provides the ability to import any of the OpenEye Python toolkits directly into the interpreter. Once a toolkit has been imported, its entire functionality is available for use within VIDA. A valid license for the imported toolkit is required in order for the import to succeed. An exception will be thrown at import time if a valid license cannot be found. All of the toolkits come directly bundled with the application, so there is no additional need to perform any installation.
Molecule, grid, and surface objects can be passed back and forth between the
main application and the Python interpreter using a handful of methods. For
stability reasons, copies of these objects must be “checked out” of VIDA,
where they can be accessed by the toolkits. If any modifications are made to
the objects in the interpreter, these modifications can be applied to the
associated objects in VIDA by “checking in” the modified object. One
exception to this rule, is that it is possible to make display changes to the
original objects by calling the built-in API functions and passing in the
unique key obtained from the object using the GetKey()
method. A number of
examples follow.
OEChem Objects¶
Most of the standard scripting functions in VIDA that operate on atoms,
bonds, or molecules take a unique key as a parameter. VIDA
adds a GetKey()
function to every OEChem object (e.g., OEMol, OESurface,
OEAtomBase, etc.) that is accessed from VIDA.
In addition, VIDA adds the following methods to all
accessed objects for convenience:
IsActive()
IsLocked()
IsMarked()
IsSelected()
IsVisible()
SetLocked( value = True )
SetMarked( value = True )
SetSelected( value = True )
SetVisible( value = True )
Access to a given molecule is provided through the
MoleculeGet
function. The molecule object returned is a copy of the actual
molecule in use in VIDA. However, this copy can still be used to retrieve
information about that original molecule as well as to alter the original
molecule’s display and associated data through the standard VIDA API.
Customizing the User Interface¶
The standard VIDA scripting API provides a wide variety of options to customize both the menus and toolbars and is detailed extensively in the appendix.
More advanced customization and extension of the user interface is possible through the PySide2 module, which provides Python wrappers for the Qt widget library used by VIDA for its user interface. Using this module within VIDA enables the user to create and interact with entirely new user interface elements. The specific details of the PySide2 module are described in the section below. For more details on Qt, please visit these links: Qt Company, The Qt Project and Qt for Python project.
PySide2¶
PySide2 is developed by the Qt for Python project and is supported by the Qt Company.
PySide2 is a complete wrapper around the Qt library which enables complex user interface customization and development.
In addition, to a complete and fully functional API for building widgets, PySide2 can also build widgets using the output from Qt Designer. Qt Designer is a graphical tool for designing and building user interface elements from Qt components. Designer can save the finished widget design to an XML file (.ui) which can then be passed to a widget object for creation in Python. The user must have a valid Qt license in order to use Qt Designer.
For more details on PySide2, please visit: Qt for Python project